NodeJS has been within the trade for some time now. Because of its asynchronous nature and assist of the Chrome V8 engine, it has change into broadly standard.
NodeJS affords quite a few frameworks that include completely different libraries, instruments, and templates to assist builders get round obstacles when creating apps.
Nodejs might be among the best JavaScript frameworks to develop a full-stack software. When you’ve determined to go along with Nodejs, the following frameworks and plugins can be helpful in creating backend and API providers.
Specific
Specific is without doubt one of the hottest net and API growth framework for NodeJS. It has been so broadly used that just about each Net growth challenge begins with the combination of Specific JS.
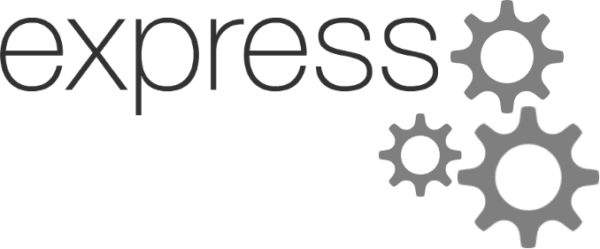
There are a bunch of causes for selecting ExpressJS as the primary plugin.
- Giant bundle of options to assist all you want in your growth duties
- Straightforward routing facility for routing your net requests to the operate
- Offers an organized platform for coding APIs
- Supported with a lot of the different supporting libraries and plugins
- Secured and maintained persistently to maintain up with the requirements
- Nice neighborhood assist
Along with these advantages, the builders of the plugin have additionally created an easy-to-use challenge generator. This generator can create a template challenge to get you off the toes sooner.
Setup Undertaking
Let’s arrange a challenge to study the fundamentals of the categorical. Be sure to have put in the node in your system. You’ll be able to consult with this definitive information for the node set up course of.
- Create a challenge folder.
- Open it within the terminal.
- Set up the
categorical
,body-parser
,cookie-parser
,cors
, andnodemon
. - Add the index.js file to the challenge.
- Add the appliance begin script within the
packages.json
file withnodemon index.js
command.
Packages
- categorical: that is our software’s core bundle which helps us create APIs.
- body-parser: it is a middleware that parses the incoming information from the API and provides it to the
req.physique
. - cookie-parser: it is a middleware that parses the header Cookie and provides it to the
req.cookie
. - cors: it is a middleware that’s used to allow the CORS.
- nodemon: that is used to run our software which is able to restart the server each time any file modifications.
These are the fundamental packages required for the categorical software to make our lives simpler. Chances are you’ll want extra packages based mostly in your challenge. Don’t fear about it now, including packages is only a command away.
Specific App
Let’s see the fundamental software with completely different APIs
const categorical = require("categorical");
const bodyParser = require("body-parser");
const cookieParser = require("cookie-parser");
const cors = require("cors");
// initializing the categorical
const app = categorical();
const port = 3000;
// including the middlewares to the app
app.use(bodyParser.json());
app.use(cookieParser());
app.use(cors());
// req: we'll use this paramter to get API request particulars
// res: we'll use
app.get("/", (req, res) => {
return res.ship("Hiya, World!");
});
app.get("/json", (req, res) => {
return res.json({ greetings: "Hiya, World!" }); // you can too use res.ship({})
});
app.get("/path-params/:title", (req, res) => {
// all the trail params will current in req.params object
const { title } = req.params;
return res.json({ greetings: `Hiya, ${title}!` });
});
app.get("/query-params", (req, res) => {
// all of the question params will current in req.question object
const { title } = req.question;
return res.json({ greetings: `Hiya, ${title ? title : "Geekflare"}!` });
});
app.put up("/put up", (req, res) => {
// information will current in req.physique
const { title } = req.physique;
console.log(req.physique);
return res.json({ greetings: `Hiya, ${title ? title : 'Geekflare'}!` });
});
app.pay attention(port, () => {
console.log(`App listening on port ${port}`);
});
Begin the appliance with npm begin
and check out all of the APIs that we have now written. Learn the documentation to study extra about every idea.
Sails
Sails is a full-fledged MVC structure framework. It makes use of ExpressJS and SocketIO at its core. Sails.js received standard for its enterprise-grade structure that allowed sooner integration with the database utilizing mannequin objects.
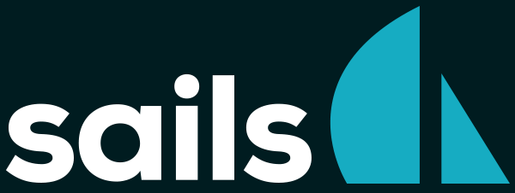
A number of the advantages are:
- Sails.JS comes with a challenge to instantly generate a challenge template
- The folder construction in Sails.JS is extraordinarily well-organized
- Growth of object fashions and exposing them utilizing frontend is speedy
- Permits straightforward integration of middleware for authorization, authentication and pre-processing
- Comes with built-in assist for AWS S3 and GridFS
Undertaking Setup
To create a sailsjs
challenge, we want an npm bundle known as sail
. Let’s set up it globally with the next command.
npm set up sails -g
Go to the listing the place you wanna create your challenge. Now, run the next command to create sailsjs
app.
sails new basic-app
A immediate will seem to pick out the template. Choose the Empty choice. Wait until it’s carried out creating the challenge.
Open the challenge in your favourite code editor. Run the sails carry
command to run the appliance. Open http://localhost:1337/
URL in your browser to see the appliance.
If you happen to see the appliance within the code editor, you can find lots of folders and information. You’ll find an in depth clarification of each folder and file, on Sails documentation web page. On this tutorial, we can be seeing one api/controllers
and config/routes.js
.
SailsJS App
Let’s see tips on how to create APIs in sailsjs app. Examine the under steps to create an API in sailsjs app.
- Add API endpoint to the
config/routes.js
file. - Create motion for the API endpoint utilizing the command
sails generate motion pattern --no-actions2
. - Add your API code within the motion file that’s generated in step 2.
Routes
The routes.js
file will look much like the next code after you add API endpoints.
module.exports.routes = {
"GET /": { motion: "dwelling" },
"GET /json": { motion: "json" },
"GET /path-params/:title": { motion: "path-params" },
"GET /query-params": { motion: "query-params" },
"POST /put up": { motion: "put up" },
};
Every API endpoint is pointing to 1 motion. We’ve to generate these motion information with the command talked about within the earlier part. Let’s generate all of the motion information for the above endpoints.
Actions
We’ve added 5 endpoints. Let’s verify the respective code for every endpoint.
dwelling.js
module.exports = async operate dwelling(req, res) {
return res.ship("Hiya, World!");
};
json.js
module.exports = async operate json(req, res) {
return res.json({ greetings: "Hiya, World!" });
};
path-params.js
module.exports = async operate pathParams(req, res) {
const { title } = req.params;
return res.json({ greetings: `Hiya, ${title}!` });
};
put up.js
module.exports = async operate put up(req, res) {
const { title } = req.physique;
console.log(req.physique);
return res.json({ greetings: `Hiya, ${title ? title : 'Geekflare'}!` });
};
query-params.js
module.exports = async operate queryParams(req, res) {
const { title } = req.question;
return res.json({ greetins: `Hiya, ${title ? title : "Geekflare"}!` });
};
There may be one other means of writing actions. Learn the documentation to study extra in regards to the framework.
Hapi
Hapi framework was initially constructed to beat the drawbacks of ExpressJS framework. Walmart sighted these drawbacks whereas they have been getting ready for a heavy site visitors occasion.
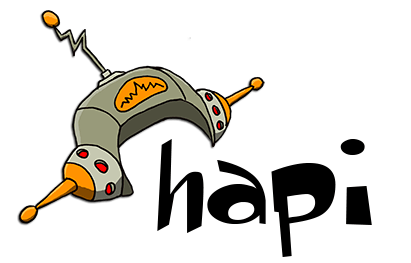
Hapi.JS is a sturdy framework to construct providers and API. It’s recognized for its stability and reliability.
Undertaking Setup
Let’s create a challenge to the touch the fundamentals of Hapi.JS. We will arrange the Hapi challenge much like regular NodeJS challenge. Run the under instructions to arrange the challenge.
cd your_project_floder_path
npm init -y ## to init node challenge
npm set up @hapi/hapi ## putting in core bundle to work with Hapi.JS
npm set up nodemon ## to run our software
Create index.js
file within the challenge. Add the beginning script within the bundle.json
file with nodemon index.js
command.
HapiJS App
Examine the fundamental APIs in Hapi under.
const hapi = require("@hapi/hapi");
const app = async () => {
// initializing the hapi server
const server = hapi.server({
port: 3000,
host: "localhost",
});
server.route({
methodology: "GET",
path: "/",
handler: (request, h) => {
return "Hiya, World!";
},
});
server.route({
methodology: "GET",
path: "/json",
handler: (request, h) => {
return { greetings: "Hiya, World!" };
},
});
server.route({
methodology: "GET",
path: "/path-params/{title}",
handler: (request, h) => {
const title = request.params.title;
return { greetings: `Hiya, ${title}!` };
},
});
server.route({
methodology: "GET",
path: "/query-params",
handler: (request, h) => {
const title = request.question.title;
return { greetings: `Hiya, ${title ? title : "Geekflare"}!` };
},
});
server.route({
methodology: "POST",
path: "/put up",
handler: (request, h) => {
const information = request.payload;
console.log(information);
return { greetings: `Hiya, ${information.title ? information.title : "Geekflare"}!` };
},
});
// beginning the server
await server.begin();
console.log(`App is working on ${server.data.uri}`);
};
app();
We’ve added completely different APIs to study the fundamentals of Hapi. You’ll be able to transfer all of the routes right into a separate file to make them clear. Go to the documentation to study extra issues about Hapi.
Whole
Whole is a server-side platform that gives a ready-to-use platform to construct real-time, chatbot, IoT, eCommerce, REST functions. It additionally permits premium customers to publish their functions on the platform for others to make use of.
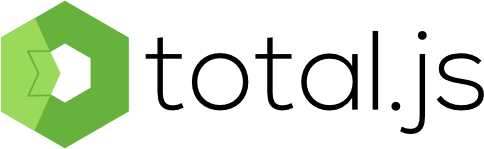
The advantages of utilizing Whole.JS as a base on your growth are:
- Fast prototyping talents
- Comes lots of pre-built elements that permits sooner growth
- Holds a library of functions that may be simply fetched and built-in into your software
- Module based mostly framework that permits simplifying work distribution in a big challenge
- Group Chat
- Persistently maintained a retailer of functions which can be prepared to be used
LoopBack
LoopBack is an API growth framework that comes built-in with API explorer. The API explorer might be related simply to client-side functions utilizing available LoopbackJS SDKs. The SDKs can be found for Android, AngularJS, Angular 2+ in addition to iOS functions.

LoopBack is trusted by GoDaddy, Symantec, Financial institution of America and plenty of extra. One can find many examples on their web site to create backend API, safe REST API, persist information, and so on. And sure, it received built-in API explorer.
Undertaking Setup
Let’s arrange the loopback challenge and see tips on how to create fundamental APIs with it.
Run the next command to put in the loopback CLI.
npm set up -g @loopback/cli
Run the under command to begin the challenge set-up.
lb4 app
Reply all of the questions within the terminal. You’ll be able to reply the questions based mostly in your desire.
Open the app in your favourite code editor and run it with npm
begin command. Go to http://localhost/
to verify the loopback app.
LoopBack App
If you happen to verify contained in the src
folder, there can be a controllers
. That is the place the place we’ll add controllers which is able to include our APIs.
We’ve to make use of the next command to create controllers in loopback.
lb4 controller
Examine the completely different APIs under. We’ve added a number of APIs to the controller.
import {get, param, put up, requestBody} from '@loopback/relaxation';
interface Response {
greetings: string;
}
export class GeekflareController {
@get('/howdy')
dwelling(): string {
return 'Hiya, World!';
}
@get('/json')
json(): Response {
return {greetings: 'Hiya, World!'};
}
// accessing path param utilizing @param decorator
@get('/path-params/{title}')
pathParams(@param.path.string('title') title: string): Response {
return {greetings: `Hiya, ${title}!`};
}
@get('/query-params')
queryParams(@param.question.string('title') title: string): Response {
return {greetings: `Hiya, ${title ? title : "Geekflare"}!`};
}
// accessing path param utilizing @requestBody decorator
@put up('/put up')
postMethod(@requestBody() information: any): Response {
console.log(information);
const {title} = information;
return {greetings: `Hiya, ${title ? title : 'Geekflare'}!`};
}
}
We’ve seen tips on how to create APIs and entry various things required for the fundamentals of REST APIs. There may be lot greater than this within the LoopBack framework. Their documentation is the best place to dive deep into the framework.
Meteor
Meteor is an entire net growth and API creation resolution with an unimaginable design at its core. Meteor is a framework that’s used for speedy software constructing. Meteor structure lets you execute code on the frontend in addition to backend with out having to re-write the code.
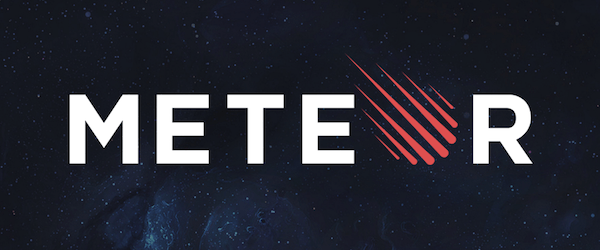
This improves the event velocity by a fantastic extent. Important advantages of utilizing Meteor are:
- Hybrid software growth framework
- With a single code base, you possibly can construct a desktop app, net app in addition to a cell software
- It comes with a tightly coupled frontend which helps in lowering your code footprint
- Extremely extensible with a spread of plugins
- Helps numerous frontend templating frameworks
- Helps scorching code push which permits eradicating the necessity for updating cell functions
Restify
Construct a production-ready semantically appropriate RESTfull net service with Restify.

It makes use of solely related Specific JS modules which make the codebase lighter in comparison with different frameworks. Trusted by Netflix, Pinterest, Joyent, and so on. – you gained’t go improper in selecting them.
Undertaking Setup
Let’s arrange the restify challenge and see tips on how to write fundamental APIs. Run the next instructions to create a brand new restify challenge.
Earlier than persevering with, be sure you have node with model 11. Restify doesn’t assist the most recent variations of node.
cd your_project_folder
npm init -y ## initializing node challenge
npm i restify ## putting in core bundle
npm i restify-plugins ## so as to add some parsing middlewares to our software
npm i nodemon ## to run our software
After putting in all of the packages, add the beginning script within the bundle.json
file with nodemon index.js
command. Don’t neglect so as to add index.js
file to the challenge.
Restify App
Let’s create some APIs to study the fundamentals.
const restify = require("restify");
const restifyPlugins = require("restify-plugins");
operate dwelling(req, res, subsequent) {
res.ship("Hiya, World!");
subsequent();
}
operate json(req, res, subsequent) {
res.json({ greetings: "Hiya, World!" }); // you can too use req.ship(JSONData)
subsequent();
}
operate pathParams(req, res, subsequent) {
const { title } = req.params;
res.json({ greetings: `Hiya, ${title}!` });
subsequent();
}
operate queryParams(req, res, subsequent) {
const { title } = req.question;
res.json({ greetings: `Hiya, ${title ? title : "Geekflare"}!` });
subsequent();
}
operate put up(req, res, subsequent) {
const information = req.physique;
console.log(information);
res.json({ greetings: `Hiya, ${information.title ? information.title : "Geekflare"}!` });
subsequent();
}
// creating restify server
const server = restify.createServer();
// including parsing middlewares
server.use(restifyPlugins.jsonBodyParser({ mapParams: true }));
server.use(restifyPlugins.queryParser({ mapParams: true }));
// including routes
server.get("/", dwelling);
server.get("/json", json);
server.get("/path-params/:title", pathParams);
server.get("/query-params", queryParams);
server.put up("/put up", put up);
// beginning the app
server.pay attention(3000, operate () {
console.log(`App is working at ${server.url}`);
});
Try the restify documentation to study extra in regards to the framework.
Koa
Koa primarily leverages code turbines to permit builders to hurry up their growth. It comes with numerous middlewares and plugins that will help you handle classes, request, cookies in addition to information transactions.
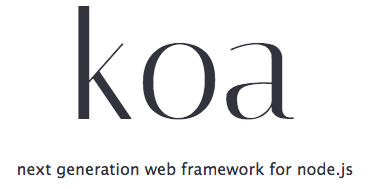
The identical workforce behind Specific designs koa. It really works with Nodejs 7.6+ and has lots of examples so that you can get it began.
Undertaking Setup
Let’s arrange the koa challenge with the next instructions
cd your_project_folder
npm init -y ## initializing the node challenge
npm i koa ## core koa bundle
npm i koa-route ## route bundle for dealing with API routes
npm i koa-bodyparser ## parser bundle to parse the request physique
npm i nodemon ## to run
Koa App
Creating APIs with koa is simple much like different frameworks that we have now seen earlier. Let’s have a look at the code.
const koa = require("koa");
const route = require("koa-route");
const bodyParser = require("koa-bodyparser");
const app = new koa();
const dwelling = async (ctx) => {
ctx.physique = "Hiya, World!";
};
const json = async (ctx) => {
ctx.physique = { greetings: "Hiya, World!" };
};
// all path parameters will will pased to the operate with the identical title that is supplied within the route
const pathParams = async (ctx, title) => {
ctx.physique = { greetings: `Hiya, ${title}!` };
};
const queryParams = async (ctx) => {
const title = ctx.question.title;
ctx.physique = { greetings: `Hiya, ${title ? title : "Geekflare"}!` };
};
const put up = async (ctx) => {
const {
physique: { title },
} = ctx.request;
ctx.physique = { greetings: `Hiya, ${title ? title : "Geekflare"}!` };
};
app.use(bodyParser());
app.use(route.get("/", dwelling));
app.use(route.get("/json", json));
app.use(route.get("/path-params/:title", pathParams));
app.use(route.get("/query-params", queryParams));
app.use(route.put up("/put up", put up));
app.pay attention(3000);
You can begin exploring the koa docs now.
Nest
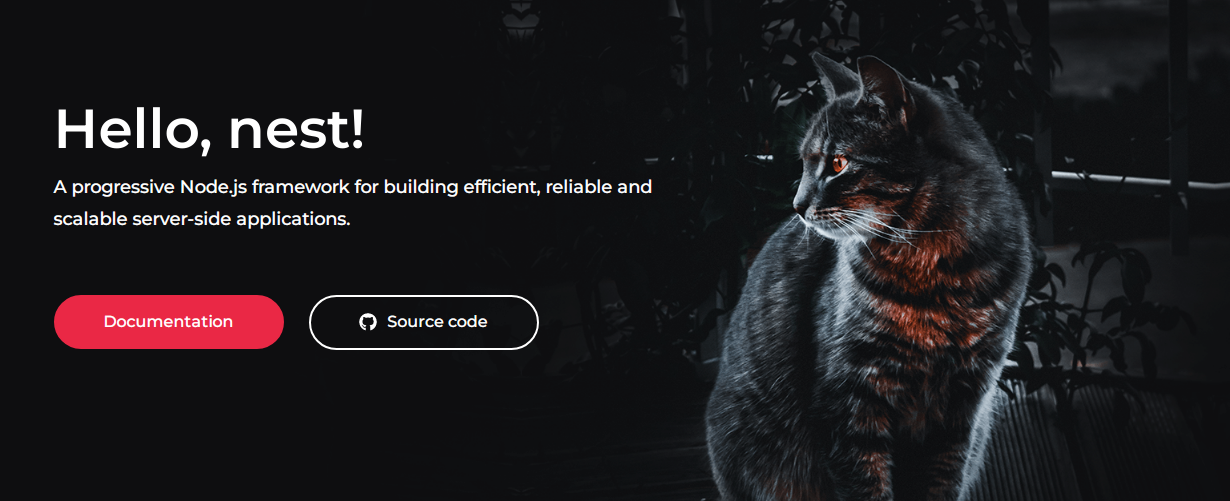
Nest is a framework for constructing server-side node functions. It makes use of categorical below the hood for the HTTP server. And we are able to configure it with Fastify as effectively.
It helps TypeScript and is constructed utilizing OOP ideas, Purposeful Programming, and Purposeful Reactive Programming. Let’s create a challenge in it and see tips on how to write fundamental APIs.
Undertaking Setup
Run the next instructions to create the challenge.
npm i -g @nestjs/cli ## putting in nest CLI globally
nest new your_project_name ## making a challenge with nest CLI
Nest App
Within the software src folder, there are some information. The app.controller.ts
is the file the place we’ll embody APIs now. Keep in mind we’re solely going to see tips on how to write fundamental functions.
Let’s take a look at completely different fundamental APIs which is able to present completely different ideas of APIs.
import { Physique, Controller, Get, Param, Submit, Question } from '@nestjs/widespread';
import { AppService } from './app.service';
export interface IResponse {
greetings: string;
}
@Controller()
export class AppController {
constructor(non-public readonly appService: AppService) {}
@Get('/')
dwelling(): string {
return 'Hiya, World!';
}
@Get('/json')
json(): IResponse {
return { greetings: 'Hiya, World!' };
}
@Get('/path-params/:title')
pathParams(@Param() params): IResponse {
const { title } = params;
return { greetings: `Hiya, ${title}!` };
}
@Get('/query-params')
queryParams(@Question() params): IResponse {
const { title } = params;
return { greetings: `Hiya, ${title ? title : 'Geekflare'}!` };
}
@Submit('/put up')
put up(@Physique() physique): IResponse {
const { title } = physique;
return { greetings: `Hiya, ${title ? title : 'Geekflare'}!` };
}
}
Run the appliance and verify all of the APIs. You too can write these APIs within the service layer and entry them within the controller.
Go to the docs of nest for extra studying and perceive the framework.
Fastify
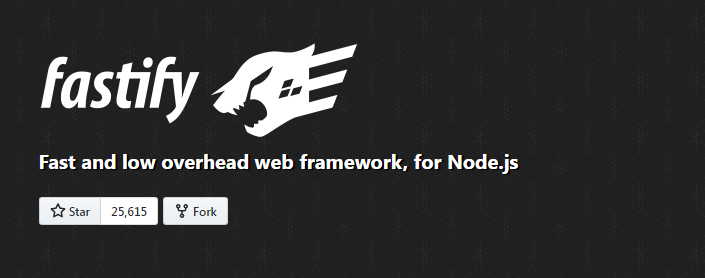
Fastify is one other framework within the NodeJS framework household. Because the title suggests, it claims it’s the one among quickest NodeJS frameworks on the market. Let’s see among the core of the framework.
- Quick and Extremely performant. It claims that it may possibly course of as much as 30K requests per second based mostly on the code complexity.
- TypeScript pleasant.
- Developer pleasant with expressive API for fast growth.
- It comes with a built-in logger. It makes use of Pino (NodeJS logger).
Undertaking Setup
Let’s arrange fastify challenge to study the fundamentals of API growth. Working the next instructions will arrange a brand new fastify challenge for you.
npm set up --global fastify-cli ## putting in fastify CLI
fastify generate project_name ## creating challenge with fastify CLI
Fastify App
Fasitfy CLI generated challenge for us. We’re presently apprehensive about solely writing APIs. To write down new APIs, open routes/root.js
file. Add the next APIs within the file.
module.exports = async operate (fastify, opts) {
fastify.get("/", async operate (request, reply) {
return "Hiya, World!";
});
fastify.get("/json", async operate (request, reply) {
return { greetings: "Hiya, World!" };
});
fastify.get("/path-params/:title", async operate (request, reply) {
const { title } = request.params;
return { greetings: `Hiya, ${title}!` };
});
fastify.get("/query-params", async operate (request, reply) {
const { title } = request.question;
return { greetings: `Hiya, ${title ? title : "Geekflare"}!` };
});
fastify.put up("/put up", async operate (request, reply) {
const { title } = request.physique;
return { greetings: `Hiya, ${title ? title : "Geekflare"}!` };
});
};
Begin the appliance and check all of the APIs. These are the fundamentals to write down the APIs. Go to the fastify docs to study extra in regards to the framework.
tinyhttp
tinyhttp is a light-weight and express-like JS framework. It comes with a built-in logger, JWT, and CORS middleware. Let’s arrange a tiny challenge and study the fundamentals of it.
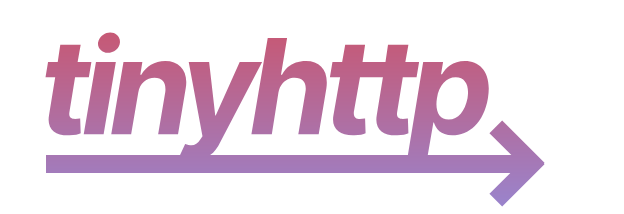
Undertaking Setup
Run the next instructions to arrange the tinyhttp challenge.
cd you_project_folder
npm init -y
npm i @tinyhttp/app ## core tinyhttp bundle
npm i milliparsec ## to parse the request information and add it to request object to physique key
npm i nodemon ## to run our software which is able to restart routinely each time information modifications
We have to do yet another factor. Add sort
key with worth module
to the bundle.json
file. We’re including it as a result of tinyhttp doesn’t assist require
, we have to use import
statements as an alternative.
tinyhttp App
The API of tinyhttp appears nearly much like categorical app. Let’s verify the completely different APIs in it.
import { App } from "@tinyhttp/app";
import { json } from "milliparsec";
const port = 3000;
const app = new App();
app.use(json());
// req: we'll use this paramter to get API request particulars
// res: we'll use
app.get("/", (req, res) => {
res.ship("Hiya, World!");
});
app.get("/json", (req, res) => {
res.json({ greetings: "Hiya, World!" }); // you can too use res.ship({})
});
app.get("/path-params/:title", (req, res) => {
// all the trail params will current in req.params object
const { title } = req.params;
res.json({ greetings: `Hiya, ${title}!` });
});
app.get("/query-params", (req, res) => {
// all of the question params will current in req.question object
const { title } = req.question;
res.json({ greetings: `Hiya, ${title ? title : "Geekflare"}!` });
});
app.put up("/put up", (req, res) => {
// information will current in req.physique
const { title } = req.physique;
res.json({ greetings: `Hiya, ${title ? title : "Geekflare"}!` });
});
app.pay attention(port, () => {
console.log(`App working on port ${port}`);
});
You’ll be able to study extra in regards to the framework of their tiny docs.
SocketIO
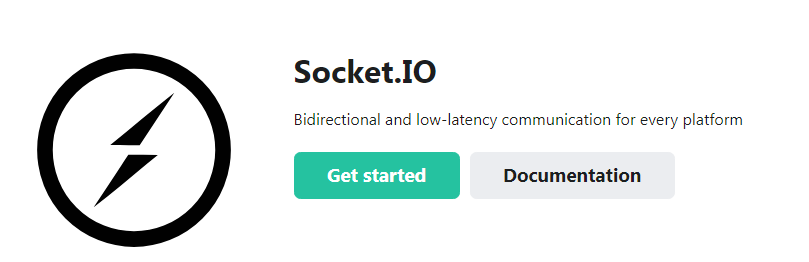
SocketIO is a web-socket framework that’s out there for a number of programming languages.
In NodeJS, SocketIO permits the constructing of net socket functions like chatbots, rating tickers, dashboard APIs and others. SocketIO has vital advantages over the traditional NodeJS net socket library.
- Help for customized URL routing for net sockets
- Auto-generated identifiers for each socket
- Straightforward administration of socket rooms to broadcast information
- Simpler integration with Specific JS
- Helps clustering with Redis
- Help for socket authentication with a further plugin – socketio-auth
- Inbuilt fallback HTTP protocol based mostly dealing with for a server which doesn’t assist HTTP 1.1
Conclusion
Individuals are utilizing NodeJS broadly throughout their tasks. Because of this, we have now numerous frameworks to select from. If you happen to see the frameworks, most of them have comparable APIs. So, if in case you have good information of NodeJS and any framework, that’s greater than sufficient to begin with every other NodeJS framework.
Creating functions shouldn’t be straightforward due to these frameworks. Make good use of the frameworks that you just like and maintain exploring others.
You might also discover some Node.js Packages to debug, write and handle their code effectively.
Blissful Coding 🙂