In case you are somebody who likes to play the snake sport, I’m certain one can find this text fascinating.
On this article, I am going to educate you find out how to give you a easy snake sport that even a newbie in Python might simply develop.
There are a variety of the way to make this sport and one contains utilizing Python’s PyGame library, which is a Python library that we use to make video games.
The opposite approach is through the use of the turtle library. This module comes pre-installed with Python and supplies customers with a digital canvas to create shapes and pictures.
Subsequently, this text will use the turtle library to implement our easy snake sport, which is beginner-friendly, particularly for novice Python builders.
Along with this module, we may also use two different modules, specifically;
- Time Module – This technique permits us to maintain observe of the variety of seconds which have handed for the reason that earlier time.
- Random module – It randomly generates numbers in Python.
Different fundamental instruments you will want embody a textual content editor of your selection. I might be utilizing VSCode on this article. After all, you will want to put in Python 3 in your pc if you happen to do not have already got it. It’s also possible to use the Geekflare compiler.
This ought to be enjoyable!
How the snake sport works
The final word goal of this sport is for the participant to get the very best rating by controlling the snake to gather the meals displayed on the display screen.
The participant controls the snake utilizing 4 directional keys relative to the course the snake is shifting. Ought to the snake hit a block or itself, the participant loses the sport.
We are going to observe the next steps to implement this sport.
- Import into our packages the pre-installed modules (tortoise, time and random).
- Creating the display screen rendering of the sport utilizing the turtle module.
- Set the keys for the snake’s course of motion throughout the display screen.
- The gameplay implementation.
Create a snakegame.py file the place we’ll add the implementation code.
Import the modules
This piece of code imports the turtle, time, and random modules that come pre-installed in Python by default. As well as, we’ll then set default values for the participant’s preliminary rating, the very best rating the participant will obtain, and the delay the participant will tackle every transfer. The time module is used right here to calculate the delay time.
Add the next piece of code to your snakegame.py file.
import turtle
import random
import time
player_score = 0
highest_score = 0
delay_time = 0.1
Create the display screen rendering of the sport
With the turtle module we’re importing right here, we will create a digital canvas that would be the window display screen of the sport. From right here we will create the snake’s physique and the meals the snake will gather. Our display screen may also show the participant’s tracked rating.
Add this code to the Python file.
# window display screen created
wind = turtle.Display()
wind.title("Snake Maze🐍")
wind.bgcolor("crimson")
# The display screen measurement
wind.setup(width=600, peak=600)
# creating the snake
snake = turtle.Turtle()
snake.form("sq.")
snake.colour("black")
snake.penup()
snake.goto(0, 0)
snake.course = "Cease"
# creating the meals
snake_food = turtle.Turtle()
shapes = random.selection('triangle','circle')
snake_food.form(shapes)
snake_food.colour("blue")
snake_food.velocity(0)
snake_food.penup()
snake_food.goto(0, 100)
pen = turtle.Turtle()
pen.velocity(0)
pen.form('sq.')
pen.colour('white')
pen.penup()
pen.hideturtle()
pen.goto(0, 250)
pen.write("Your_score: 0 Highest_Score : 0", align="heart",
font=("Arial", 24, "regular"))
turtle.mainloop()
The code snippet above begins by initializing the turtle display screen and passes a title and a background colour to the display screen. After defining the window measurement of our display screen, we draw the form of the snake on the digital canvas.
The penup() technique merely picks up the turtle’s pen, so no line is drawn because the turtle strikes. The goto(x,y) technique comprises coordinate positions that transfer the turtle to an absolute place.
Subsequent, we make the meals that the snake collects. We need to show the participant’s rating every time the snake collects the meals and the very best rating the participant achieves throughout the sport. So we use the pen.write() technique to implement this. The hideturtle() hides the turtle icon from the display screen on the header space the place this textual content is written.
You will need to add the turtle.mainloop() to the top of your code, which makes the display screen seem longer so that you simply as a consumer can do one thing on the display screen.
Run the file and it’s best to have the next output:
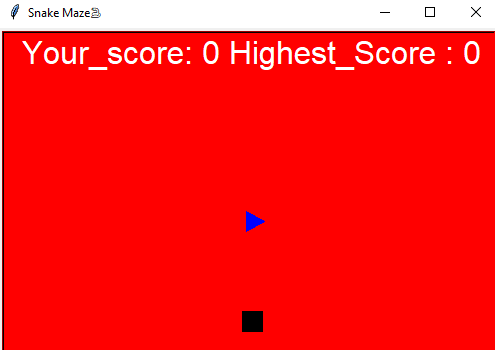
Set the directional keys for the snake
Right here we’ll arrange particular keys that decide the course the snake will transfer on the display screen. We use the ‘L’ for left, ‘R’ for proper, ‘U’ for up, ‘D’ for down. We are going to implement these instructions utilizing the turtle’s course perform which we’ll name on the snake.
Add the next code snippet to your code.
# Assigning instructions def moveleft(): if snake.course != "proper": snake.course = "left" def moveright(): if snake.course != "left": snake.course = "proper" def moveup(): if snake.course != "down": snake.course = "up" def movedown(): if snake.course != "up": snake.course = "down" def transfer(): if snake.course == "up": coord_y = snake.ycor() snake.sety(coord_y+20) if snake.course == "down": coord_y = snake.ycor() snake.sety(coord_y-20) if snake.course == "proper": coord_x = snake.xcor() snake.setx(coord_x+20) if snake.course == "left": coord_x = snake.xcor() snake.setx(coord_x-20) wind.pay attention() wind.onkeypress(moveleft, 'L') wind.onkeypress(moveright, 'R') wind.onkeypress(moveup, 'U') wind.onkeypress(movedown, 'D')
The transfer() perform above units the motion of the snake within the outlined place inside a exact coordinate worth.
The pay attention() perform is an occasion listener that calls the strategies that transfer the snake in a sure course when the participant presses the important thing.
The snake sport gameplay implementation
After capturing the fundamental views of our snake sport, we’ll must make the sport real-time.
This implies:
- Let the snake develop in size every time it collects the meals by ideally utilizing a distinct colour.
- Enhance the participant’s rating every time the snake collects the meals and observe the very best rating.
- The participant can stop the snake from crashing into the wall or his personal physique.
- The sport begins once more when the snake collides.
- The participant’s rating is reset to zero when the sport is restarted, whereas the display screen retains the participant’s highest rating.
Add the remainder of this code to your Python file.
segments = []
#Implementing the gameplay
whereas True:
wind.replace()
if snake.xcor() > 290 or snake.xcor() < -290 or snake.ycor() > 290 or snake.ycor() < -290:
time.sleep(1)
snake.goto(0, 0)
snake.course = "Cease"
snake.form("sq.")
snake.colour("inexperienced")
for section in segments:
section.goto(1000, 1000)
segments.clear()
player_score = 0
delay_time = 0.1
pen.clear()
pen.write("Participant's_score: {} Highest_score: {}".format(player_score, highest_score), align="heart", font=("Arial", 24, "regular"))
if snake.distance(snake_food) < 20:
coord_x = random.randint(-270, 270)
coord_y = random.randint(-270, 270)
snake_food.goto(coord_x, coord_y)
# Including section
added_segment = turtle.Turtle()
added_segment.velocity(0)
added_segment.form("sq.")
added_segment.colour("white")
added_segment.penup()
segments.append(added_segment)
delay_time -= 0.001
player_score += 5
if player_score > highest_score:
highest_score = player_score
pen.clear()
pen.write("Participant's_score: {} Highest_score: {}".format(player_score, highest_score), align="heart", font=("Arial", 24, "regular"))
# checking for collisions
for i in vary(len(segments)-1, 0, -1):
coord_x = segments[i-1].xcor()
coord_y = segments[i-1].ycor()
segments[i].goto(coord_x, coord_y)
if len(segments) > 0:
coord_x = snake.xcor()
coord_y = snake.ycor()
segments[0].goto(coord_x, coord_y)
transfer()
for section in segments:
if section.distance(snake) < 20:
time.sleep(1)
snake.goto(0, 0)
snake.course = "cease"
snake.colour('white')
snake.form('sq.')
for section in segments:
section.goto(1000, 1000)
section.clear()
player_score = 0
delay_time = 0.1
pen.clear()
pen.write("Participant's_score: {} Highest_score: {}".format(player_score, highest_score), align="heart", font=("Arial", 24, "regular"))
time.sleep(delay_time)
turtle.mainloop()
Within the code snippet above, we set a random place for the snake’s meals on the display screen. Every time the snake collects this meals, its physique section will increase by a distinct colour; white, on this case, to tell apart the expansion.
After the snake collects the meals with out colliding, the meals is positioned at a random place inside the 270 coordinate vary of the display screen measurement. Every time the snake collects meals, the participant’s rating is elevated by 5. When the snake collides, the participant’s rating is reset to 0 whereas the display screen retains the very best rating.
Now return the Python file and it’s best to see your turtle display screen appear like this:
Conclusion 🐍
Utilizing the turtle library is a enjoyable and straightforward solution to create the snake sport, as we have seen on this article. Alternatively, you’ll be able to implement the identical utilizing the PyGame library. You’ll be able to take a look at the PyGame tutorial right here and see how one can implement the sport in another way.
It’s also possible to strive a guessing sport in Python or find out how to get JSON information in Python.
Have enjoyable coding!