This tutorial will train you ways to do this print Pascal’s triangle in Python for a given variety of rows.
You begin by studying to assemble Pascal’s triangle. You then transfer on to writing a Python operate and learn to optimize it additional.
▶️ Let’s get began!
What’s Pascal’s triangle and the right way to assemble it?
Printing Pascal’s triangle for a given variety of rows is a well-liked interview query.
In Pascal’s triangle with N rows, row quantity i has i parts.
So the primary row has one ingredient, and it’s 1. And every ingredient in subsequent rows is the sum of the 2 numbers straight above It.
The next determine explains the right way to assemble Pascal’s triangle with 5 rows.
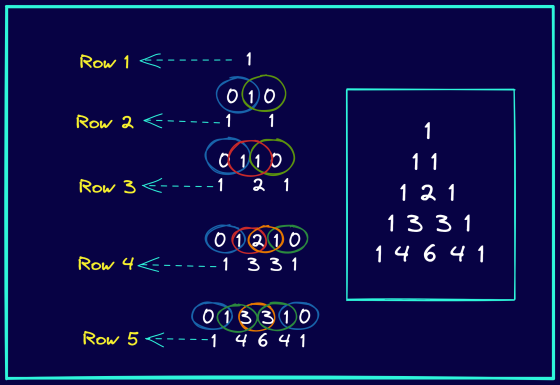
Be aware the right way to fill in zeroes when you’ve got any just one quantity above a sure quantity.
📝As a fast train, observe the process above to assemble Pascal’s triangle for n = 6 and n = 7.
Subsequent, let’s transfer on to writing some code. You possibly can select to run the code snippets on Geekflare’s Python IDE proper out of your browser – as you’re employed your approach via the tutorial.
Python operate to print Pascal’s triangle
On this part, let’s write a Python operate to print Pascal’s triangle for any variety of rows.
There are two vital questions to think about:
- Tips on how to specific the entries in Pascal’s triangle?
- Tips on how to print Pascal’s triangle with correct spacing and formatting?
Let’s reply them now.
#1. What’s the expression for every merchandise in Pascal’s triangle?
Coincidentally, the entries in Pascal’s triangle could be obtained utilizing the system for nCr
. When you keep in mind out of your college math, nCr
signifies the variety of methods you possibly can select r
gadgets from a set of n
articles.
The system for nCr
is given under:
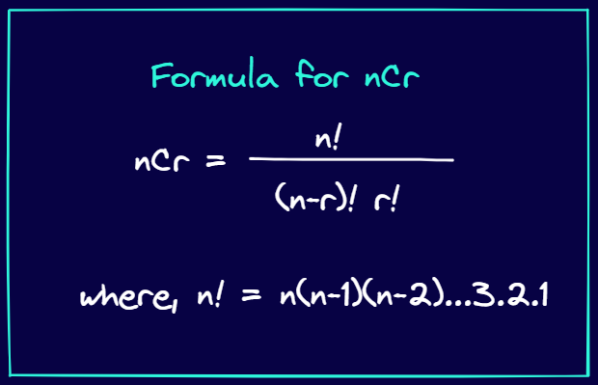
Now let’s transfer on to expressing the entries in Pascal’s triangle utilizing the nCr
system.
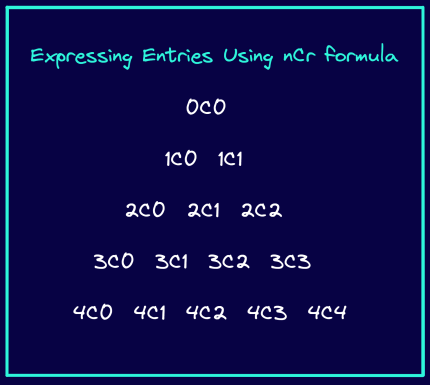
We now have now discovered a option to specific the enter within the matrix.
#2. Tips on how to modify the spacing when printing the sample?
In Pascal’s triangle with numRows
, row #1 has one entry, row #2 has two entries, and so forth. To print the sample as a triangle you will want numRows - i
areas in row #i. And you need to use Python vary
operate together with for
loop to do that.
Because the
vary
operate excludes the endpoint by default, make sure that so as to add+ 1
to get the required variety of main areas.
Now that you have realized the right way to symbolize inputs and likewise the right way to modify house whereas printing Pascal’s triangle, let’s transfer on and outline the operate pascal_tri
.
Parse the operate definition
So what would you like the operate pascal_tri
To do?
- The operate
pascal_tri
should settle for the variety of rows (numRows
) as an argument. - It ought to print Pascal’s triangle with
numRows
.
Let’s use to calculate the factorial factorial
operate of Python’s built-in math
module.
▶️ Run the next code cell to import factorial
and use it in your present module.
from math import factorial
The code snippet under accommodates the operate definition.
def pascal_tri(numRows):
'''Print Pascal's triangle with numRows.'''
for i in vary(numRows):
# loop to get main areas
for j in vary(numRows-i+1):
print(finish=" ")
# loop to get parts of row i
for j in vary(i+1):
# nCr = n!/((n-r)!*r!)
print(factorial(i)//(factorial(j)*factorial(i-j)), finish=" ")
# print every row in a brand new line
print("n")
The operate works as follows:
- The operate
pascal_tri
has one required parameternumRows
: the variety of rows. - There are
numRows
rows in complete. For every rowi
we addnumRows - i
main areas for the primary merchandise within the row. - Then we use
nCr
system to calculate the person gadgets. For rowi
the entries areiCj
The placej = {0,1,2,..,i}
. - Be aware that we use
//
which performs division by integers, as a result of we would like the inputs to be integers. - After you calculate all of the inputs in a row, print the subsequent row on a brand new line.
🔗 Since we added a docstring, you need to use the built-in Python assist
operate, or the __doc__
attribute to entry the operate’s docstring. The code snippet under reveals you ways to do that.
assist(pascal_tri)
# Output
Assistance on operate pascal_tri in module __main__:
pascal_tri(numRows)
Print Pascal's triangle with numRows.
pascal_tri.__doc__
# Output
Print Pascal's triangle with numRows.
Now let’s go forward and name the operate with the variety of rows as an argument.
pascal_tri(3)
# Output
1
1 1
1 2 1
As anticipated, the primary 3 rows of Pascal’s triangle are printed.
Print Pascal’s triangle utilizing recursion
Within the earlier part, we recognized the mathematical expression of every merchandise within the Pascal triangle. Nevertheless, we didn’t use the connection between entries in two consecutive rows.
In actual fact, we used the earlier row to calculate the enter within the subsequent row. Cannot we use this and a recursive execution of the operate pascal_tri
?
Sure lets do this!
In a recursive implementation calls a operate repeatedly till the foremost factor is fulfilled. Within the development of Pascal’s triangle, we begin with the primary row with one entrance
1
after which construct the next rows.
So the operate calls to pascal_tri(numRows)
calls in flip pascal_tri(numRows-1)
and so forth, as much as the bottom case pascal_tri(1)
has been reached.
Contemplate the instance the place you must print the primary 3 rows of Pascal’s triangle. The next picture explains how the recursive calls are pushed to the stack. And the way the recursive operate calls return the rows of Pascal’s triangle.
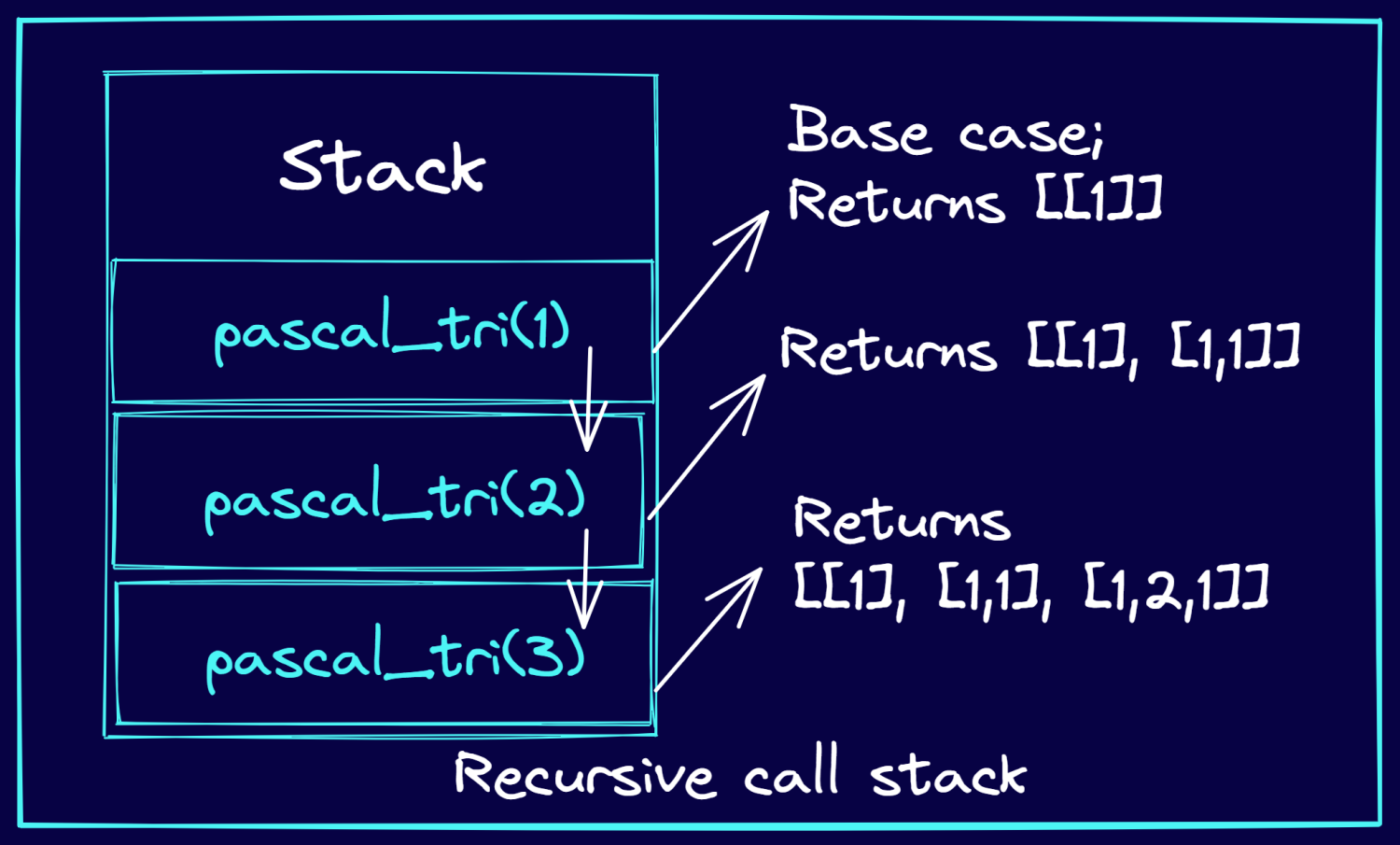
▶️ Run the code snippet under to recursively generate the rows of Pascal’s triangle.
def pascal_tri(numRows):
'''Print Pascal's triangle with numRows.'''
if numRows == 1:
return [[1]] # base case is reached!
else:
res_arr = pascal_tri(numRows-1) # recursive name to pascal_tri
# use earlier row to calculate present row
cur_row = [1] # each row begins with 1
prev_row = res_arr[-1]
for i in vary(len(prev_row)-1):
# sum of two entries instantly above
cur_row.append(prev_row[i] + prev_row[i+1])
cur_row += [1] # each row ends with 1
res_arr.append(cur_row)
return res_arr
Listed here are a number of factors value noting:
- We used a nested checklist as a knowledge construction, the place every row in Pascal’s triangle is a listing of its personal, like this: [[row 1], [row 2],…,[row n]].
- The operate name
pascal_tri(numRows)
triggers a sequence of recursive calls withnumRows - 1
,numRows - 2
all the best way to 1 because the arguments. These calls are pushed onto a stack. - When
numRows == 1
we have now reached the bottom case and the operate returns [[1]]. - Now the returned checklist is utilized by the next features within the name stack to calculate the subsequent row.
- If
cur_row
is the present row,cur_row[i] = prev_row[i] + prev_row[i+1]
—the sum of two parts instantly above the present index.
Because the returned array is a nested checklist (checklist of lists), we have to modify the spacing and print the entries as proven within the code cell under.
tri_array = pascal_tri(5)
for i,row in enumerate(tri_array):
for j in vary(len(tri_array) - i + 1):
print(finish=" ") # main areas
for j in row:
print(j, finish=" ") # print entries
print("n") # print new line
The output is appropriate, as proven under!
# Output
1
1 1
1 2 1
1 3 3 1
1 4 6 4 1
Python operate to print Pascal’s triangle for variety of rows ≤ 5
Each of the strategies you realized work to print Pascal’s triangle for any variety of rows numRows
.
Nevertheless, there are occasions when you must print Pascal’s triangle for a smaller variety of rows. And when the variety of rows you must print is as much as 5, you need to use a easy approach.
Undergo the determine under. And look how the powers of 11 are an identical to the entries in Pascal’s triangle. Additionally word that this solely works to the 4th energy of 11. That’s, 11 raised to the powers {0, 1, 2, 3, 4} provides the entries in rows 1 via 5 of Pascal’s triangle.
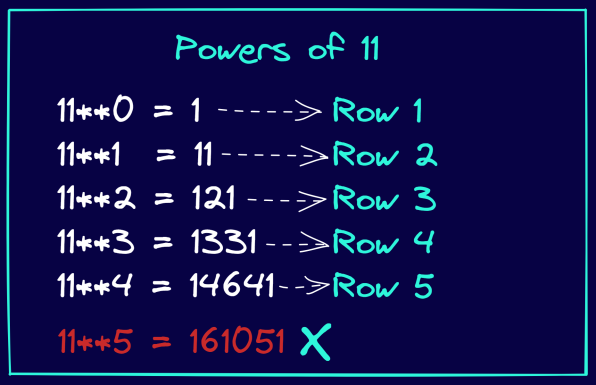
Let’s rewrite the operate definition as proven under:
def pascal_tri(numRows):
'''Print Pascal's triangle with numRows.'''
for i in vary(numRows):
print(' '*(numRows-i), finish='')
# compute energy of 11
print(' '.be a part of(str(11**i)))
Here is how the operate pascal_tri
to work:
- As with the earlier examples, we modify the spacing.
- After which we use Python’s exponentiation operator (**) to calculate the powers of 11.
- Because the powers of 11 are integers by default, convert them to a string utilizing str(). You now have the powers of 11 as strings.
- Strings in Python are iterables, so you possibly can loop via them and entry one character at a time.
- Then you need to use the
be a part of()
technique with the syntax:<sep>.be a part of(<iterable>)
merge parts<iterable>
utilizing<sep>
as a separator. - Right here you will have one single house between characters
<sep>
might be' '
,<iterable>
is string: energy of 11.
Let’s have a look at if the characteristic works as meant.
pascal_tri(5)
# Output
1
1 1
1 2 1
1 3 3 1
1 4 6 4 1
As one other instance, name the operate pascal_tri
with 4 as an argument.
pascal_tri(4)
# Output
1
1 1
1 2 1
1 3 3 1
Hope you perceive the right way to simply print Pascal triangle for numRows
within the vary from 1 to five.
Conclusion
Here is what we realized:
- Tips on how to assemble Pascal’s triangle with given variety of rows. Every quantity in every row is the sum of the 2 numbers instantly above it.
- Write a Python operate utilizing the system nCr = n!/(nr)!.r! to calculate the entries of Pascal’s triangle.
- You then realized one recursive implementation of the operate.
- Lastly, you will have realized essentially the most optimum technique to preconstruct Pascal’s triangle
numRows
as much as 5 – utilizing the powers of 11.
If you wish to enhance your Python abilities, learn to multiply matrices, examine if a quantity is prime, and clear up issues with string operations. Have enjoyable coding!