Python is probably the most extensively used programming language. At this time you’ll learn to use one in every of its most necessary (however typically ignored) features, which is unpacking in Python.
You’ve got most likely seen and even used * and ** in different folks’s code with out actually figuring out what their goal is. We’ll undergo the idea of unpacking, and the way we will use it to put in writing extra Python code.
Here’s a listing of ideas that you’ll find helpful whereas studying this tutorial:
- Iterable: Any sequence that may be repeated by a for loopcomparable to units, lists, tuples, and dictionaries
- On name: A Python object that may be known as with double parentheses (), for instance my operate()
- Shell: Interactive runtime atmosphere that permits us to run Python code. We will invoke it by operating “python” in a terminal
- Variable: Symbolic identify that shops an object and has a reserved reminiscence location.
Let’s begin with the commonest confusion: asteristics in Python are additionally arithmetic operators. One star
is used for multiplication, whereas two of them (**) confer with exponentiation.
>>> 3*3
9
>>> 3**3
27
We will show that by opening a Python shell and typing:
Observe: You have to have Python 3 put in to observe this tutorial. If you do not have it put in, try our Python set up information.
As you’ll be able to see, we use the asterisk after the primary quantity and earlier than the second quantity. For those who see this, it means we’re utilizing the arithmetic operators.
>>> *vary(1, 6),
(1, 2, 3, 4, 5)
>>> {**{'vanilla':3, 'chocolate':2}, 'strawberry':2}
{'vanilla': 3, 'chocolate': 2, 'strawberry': 2}
Then again, we use the asterisks (*, **) earlier than an iterable to extract it, for instance:
Don’t be concerned in the event you do not perceive, that is simply preparation for unpacking in Python. So go forward and skim the complete tutorial!
What’s unpacking?
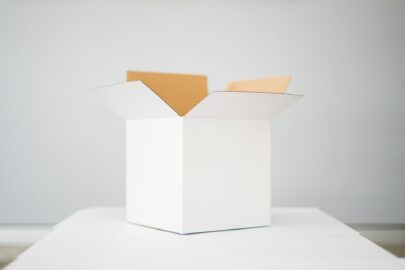
Unpacking in Python is much like unpacking a field in actual life.
>>> mybox = ['cables', 'headphones', 'USB']
>>> item1, item2, item3 = mybox
Let’s translate the identical instance into code for higher understanding: As you’ll be able to see, we level out the three objects within the my field listing of three variablesarticle1, article2, article2
. This type of variable project is the elemental idea of unpacking in Python. For those who strive to determine the worth of every merchandise, you’ll discoverentry 1 refers to “cables”,article2
>>> item1
'cables'
>>> item2
'headphones'
>>> item3
'USB'
refers to “headphones” and so forth.
>>> newbox = ['cables', 'headphones', 'USB', 'mouse']
>>> item1, item2, item3 = newbox
Traceback (most up-to-date name final):
File "<stdin>", line 1, in <module>
ValueError: too many values to unpack (anticipated 3)
To this point every part appears to be going high quality with this code, however what if we wished to extract a listing with extra components into it, maintaining the identical variety of assigned variables?
You most likely anticipated this sort of error. Primarily, we assign 4 listing objects to 3 variables. How does Python handle to assign the right values? That isn’t the case, as a result of we get a ValueError saying “too many values to extract”. This occurs as a result of we’re sitting three variables on the left, and 4
values (equivalent to the newbox listing) on the precise. For those who attempt to run an identical course of, however with extra variables than values to extract, you find yourself with a special course of Worth error
>>> lastbox = ['cables', 'headphones']
>>> item1, item2, item3 = lastbox
Traceback (most up-to-date name final):
File "<stdin>", line 1, in <module>
ValueError: not sufficient values to unpack (anticipated 3, bought 2)
besides that with a barely completely different message:
Observe: we labored with lists, however you should utilize this type of unpacking with any iterable model (lists, units, tuples, dictionaries)
So how can we overcome this example? Is there a option to extract all objects of an iterable to quite a few variables with out getting errors?
It actually is, and it is known as unpacking operator or asterisk operator (*, **). Let’s have a look at how you can use it in Python.
Extracting lists with the * operator
The asterisk operator
>>> first, *unused, final = [1, 2, 3, 5, 7]
>>> first
1
>>> final
7
>>> unused
[2, 3, 5]
is used to extract all values of an iterable that haven’t but been assigned.
>>> first, *_, final = [1, 2, 3, 5, 7]
>>> _
[2, 3, 5]
Suppose you need to retrieve the primary and final ingredient of a listing with out utilizing indexes. We will do that with the asterisk operator:
>>> first, *_, final = [1, 2]
>>> first
1
>>> final
2
>>> _
[]
As you’ll be able to perceive, we get all of the unused values with the asterisk operator. The popular option to take away values is to make use of an underline variable (_), which is usually used as a “dummy variable”.
We will nonetheless use this trick even when the listing solely comprises two components:
On this case, the underline variable (dummy variable) shops an empty listing, in order that the opposite two variables round it might probably entry the obtainable values of the listing.
>>> *string = 'PythonIsTheBest'
Basic troubleshooting We will extract a singular ingredient from an iterable. For instance, you would provide you with one thing like this:Nevertheless, the code above returns a
>>> *string = 'PythonIsTheBest'
File "<stdin>", line 1
SyntaxError: starred project goal have to be in a listing or tuple
Syntax error
:
It’s because in line with the PEP specification:
>>> *string, = 'PythonIsTheBest'
>>> string
['P', 'y', 't', 'h', 'o', 'n', 'I', 's', 'T', 'h', 'e', 'B', 'e', 's', 't']
A tuple (or listing) to the left of a easy command If we need to extract all values of an iterable variable right into a single variable, we have to arrange a tuple. Subsequently, including a easy comma is sufficient: One other instance is utilizing the
>>> *numbers, = vary(5)
>>> numbers
[0, 1, 2, 3, 4]
vary
operate, which returns an array of numbers.
Now that you know the way to unpack lists and tuples with an asterisk, it is time to unpack dictionaries.
Extract dictionaries with ** operator
>>> **greetings, = {'whats up': 'HELLO', 'bye':'BYE'}
...
SyntaxError: invalid syntax
Whereas a single asterisk is used to extract lists and tuples, the double asterisk (**) is used to extract dictionaries.
>>> meals = {'fish':3, 'meat':5, 'pasta':9}
>>> colours = {'purple': 'depth', 'yellow':'happiness'}
>>> merged_dict = {**meals, **colours}
>>> merged_dict
{'fish': 3, 'meat': 5, 'pasta': 9, 'purple': 'depth', 'yellow': 'happiness'}
Sadly, we can’t unpack a dictionary to a single variable, as now we have executed with tuples and lists. Meaning the next will throw an error:
Nevertheless, we will use the ** operator in callables and different dictionaries. For instance, if we need to create a merged dictionary, constructed from different dictionaries, we will use the code under:
It is a pretty brief means of making compound dictionaries, but it surely’s not the principle strategy to unpacking in Python.
Let’s have a look at how you can use unpacking with callables
Wrapping in features: args and kwargs
You’ve got most likely seen args and kwargs earlier than they have been carried out in courses or features. Let’s have a look at why we should always use them along with callables.
>>> def product(n1, n2):
... return n1 * n2
...
>>> numbers = [12, 1]
>>> product(*numbers)
12
Wrapping with the * operator (args) Suppose now we have a operate that calculates the product of two numbers. As you’ll be able to see we’re unpacking the listing
>>> product(12, 1)
12
Numbers
>>> numbers = [12, 1, 3, 4]
>>> product(*numbers)
...
TypeError: product() takes 2 positional arguments however 4 got
to the operate, so we’re mainly operating: Every little thing works high quality up to now, however what if we wished to cross on an extended listing? It’ll undoubtedly throw an error as a result of the operate receives extra arguments than it might probably handle. We will resolve all this simply by
>>> def product(*args):
... consequence = 1
... for i in args:
... consequence *= i
... return consequence
...
>>> product(*numbers)
144
packing up the listing straight on the operate, creating an iterable inside it and permitting us to cross any variety of arguments to the operate. Right here we cowl the
arg
parameter as an iterable, iterating by means of the weather and returning the product of all numbers. Observe that the beginning variety of the consequence have to be one, as a result of if we begin with zero, the operate will all the time return zero.
>>> product(5, 5, 5)
125
>>> print(5, 5, 5)
5 5 5
Observe: args is only a conference; you should utilize every other parameter identify We will additionally cross arbitrary numbers to the operate with out utilizing a listing, similar to the built-in print operate. Lastly, let’s outline the thing kind of the
>>> def test_type(*args):
... print(kind(args))
... print(args)
...
>>> test_type(1, 2, 4, 'a string')
<class 'tuple'>
(1, 2, 4, 'a string')
arg of a operate. As famous within the above code, the kind is argall the time can be
tuple
and its content material consists of all non-keywords handed to the operate.
Wrapping with the ** operator (kwargs) As we noticed earlier, the ** operator is used solely for dictionaries. Because of this with this operator we will cross key-value pairs as parameters to the operate.Let’s create a operate
>>> def make_person(identify, **kwargs):
... consequence = identify + ': '
... for key, worth in kwargs.objects():
... consequence += f'{key} = {worth}, '
... return consequence
...
>>> make_person('Melissa', id=12112, location='london', net_worth=12000)
'Melissa: id = 12112, location = london, net_worth = 12000, '
create_person that receives a positional argument ‘identify’ and an undefined variety of key phrase arguments. As you’ll be able to see, the
**quartz
assertion converts all key phrase arguments right into a dictionary, which we will iterate inside the operate. Observe: Kwargs is only a conference. You’ll be able to identify this parameter something you want We will verify the kind quarksthe identical means we did
>>> def test_kwargs(**kwargs):
... print(kind(kwargs))
... print(kwargs)
...
>>> test_kwargs(random=12, parameters=21)
<class 'dict'>
{'random': 12, 'parameters': 21}
arg : The
quarks inner variable all the time turns right into a dictionary, which shops the key-value pairs handed to the operate. Lastly, let’s benefit from it arg And
>>> def my_final_function(*args, **kwargs):
... print('Kind args: ', kind(args))
... print('args: ', args)
... print('Kind kwargs: ', kind(kwargs))
... print('kwargs: ', kwargs)
...
>>> my_final_function('Python', 'The', 'Greatest', language='Python', customers='Rather a lot')
Kind args: <class 'tuple'>
args: ('Python', 'The', 'Greatest')
Kind kwargs: <class 'dict'>
kwargs: {'language': 'Python', 'customers': 'Rather a lot'}
quarks
in the identical place:
Conclusion
- Unpacking operators are very helpful in on a regular basis duties, now that you know the way to make use of them, each in particular person statements and in operate parameters.
- On this tutorial you realized:
- You utilize * for tuples and lists and ** for dictionaries You should utilize unpacking operators in features and sophistication constructors
- arg are used to cross parameters with out key phrases to features
quarks
are used to cross key phrase parameters to features. Subsequent, you would possibly learn to use the Python Not Equal operators.