Check engineers are important within the software program growth lifecycle, as they make sure that a staff delivers bug-free and dealing functions. These engineers undergo a number of exams earlier than the functions are shipped or declared prepared to be used.
Testers have to be proficient and in a position to find and work together with net components. Selenium is among the most used take a look at automation instruments by fashionable growth groups. This device consists of 4 parts; Selenium Grid, Selenium WebDriver, Selenium IDE and Selenium RC.
Our focus in the present day shall be on Selenium WebDriver, because it consists of XPath. This text defines XPath, discusses fundamental XPath syntax, and illustrates how XPath works in Selenium.
What’s XPath
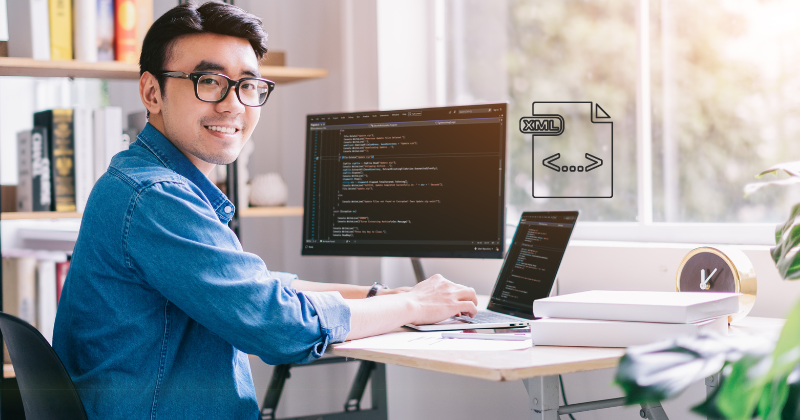
XPath (XML Path Language) is a question language for choosing and navigating attributes and components inside XML paperwork. XPath defines a path expression and supplies a strategy to deal with and retrieve info from particular elements of an XML doc.
The syntax is just like a file system path. It additionally options capabilities and symbols that make deciding on components based mostly on their attributes and hierarchy straightforward. You need to use XPath with applied sciences similar to XML, HTML, and XSLT to extract and manipulate knowledge.
Why use XPath?
- It is versatile: Not like CSS selectors which may solely localize components utilizing tag identify, ID or class, XPath means that you can localize components utilizing different attributes.
- Reusable: You possibly can retailer your XPath in variables and reuse them in your code.
- Correct node choice: XPath supplies a standardized strategy to goal particular components in an internet doc.
XPath fundamental syntax
XPath means that you can discover any aspect on an internet web page utilizing the DOM. Nevertheless, earlier than we test the syntax, we have to perceive the next XPath expressions;
Expression | Description |
node identify/tag identify | Selects all nodes named ‘nodename’/ ‘tagname’ |
/ | Selects from the basis node |
// | Selects nodes within the present doc from the present node that match the choice, no matter the place they’re positioned |
@ | Selects attributes |
.. | Selects the father or mother node of the present node |
. | Selects the present node |
The default syntax for XPath is;
XPath=//tagname[@attribute='value']
As you’ll be able to see, the syntax begins with a double slash (//), which begins with the present node as outlined by the tag/node identify.
Absolute XPath vs Relative XPath
We’ve two paths when coping with XPath; Absolute XPath and Relative XPath;
Positively XPath
An absolute XPath is a direct path from the basis to the specified aspect. You begin with the basis node and finish with the goal node.
You possibly can have an HTML doc with the next content material;
<!DOCTYPE html>
<html>
<head>
<title>Geekflare</title>
</head>
<physique>
<div>
<h1>Welcome to Geekflare</h1>
</div>
</physique>
</html>
If we need to discover the aspect with the content material “Welcome to Geekflare”, comply with this path;
/html/physique/div/h1
Within the above doc we’ve;
- The html as the basis node:
/html
- The physique is a father or mother node:
/html/physique
- The div as a baby of the physique node:
/html/physique/div
- The h1 as a baby of the div node:
/html/physique/div/h1
To succeed in the innermost aspect, you need to comply with that path.
When must you use Absolute XPath?
Absolute XPath follows a ‘particular’ path. So it is an ideal match in case you have a number of components with related traits on a web page, ensuring you are concentrating on the precise components in a doc.
Nevertheless, XPath is oversensitive to adjustments within the construction of the HTML doc. As such, a easy change can break your Absolute XPath.
Relative XPath
Relative XPath begins from every node and ends with the goal node. This path is unaffected by adjustments to the doc, making it preferable most often. Relative XPath means that you can find components from any a part of the doc. The Relative XPath expression begins with double slashes ‘//’.
If we use the HTML doc, we are able to discover our H1 that claims “Welcome to Geekflare”;
<!DOCTYPE html>
<html>
<head>
<title>Geekflare</title>
</head>
<physique>
<div>
<h1>Welcome to Geekflare</h1>
</div>
</physique>
</html>
Our relative XPath to h1 shall be;
//physique/div/h1
When must you use relative XPath?
It is best to use Relative XPath in case you are on the lookout for a steadiness between flexibility and specificity. This path is immune to adjustments within the HTML doc, as the connection between the weather stays particular.
Discover components utilizing XPath in Selenium
Selenium is an open-source framework that enables customers to automate net browsers. The framework has a group of libraries and instruments that permit testers to work together with net components routinely and systematically.
Assuming we’ve an internet doc containing an inventory of numbers as follows;
<!DOCTYPE html>
<html>
<head>
<title>Track Library</title>
</head>
<physique>
<h1>Track Library</h1>
<ul class="song-list">
<li class="music" title="Track Title 1">Track 1 - Artist 1</li>
<li class="music" title="Track Title 2">Track 2 - Artist 2</li>
<li class="music" title="Track Title 3">Track 3 - Artist 1</li>
<li class="music" title="Track Title 4">Track 4 - Artist 3</li>
</ul>
</physique>
</html>
- Our primary node is .
- We’ve as our father or mother node.
- We’ve
as a baby of .
- We’ve
- as a baby of .
- We’ve
- as a baby of
- .
We will use totally different XPath locators within the above HTML doc. For instance, we are able to find components by ID, identify, class identify, comprises, texts, ends with and begins with, amongst many different locators. You need to use Selenium with totally different programming languages. We are going to use Python to reveal.
Search by index
Assuming we need to discover quantity 3, we are able to have this code;
third_song = driver.find_element_by_xpath("//li[@class='song'][3]")
print("Third Track:", third_song.textual content)
We used Relative XPath and begin with the ‘li’ node. When Selenium finds the third quantity on our checklist, it can print its lyrics.
Search by attribute
We will have an XPath that finds all of the songs from ‘Artist 1’ and prints their titles. Our code might be as follows;
songs_by_artist1 = driver.find_elements_by_xpath("//li[contains(@class, 'song') and contains(text(), 'Artist 1')]")
print("Songs by Artist 1:")
for music in songs_by_artist1:
print(music.textual content)
Search by textual content
This locator helps you discover components with particular textual content. We will seek for a music that claims “Track 4” and print its lyrics. We will show this locator utilizing this code;
song_with_text = driver.find_element_by_xpath("//li[contains(text(), 'Song 4')]")
print("Track with Textual content:", song_with_text.textual content)
XPath axes
The approaches we have mentioned up to now work completely effectively with easy net pages. Nevertheless, there are instances the place search strategies for XPath components, similar to by Textual content, ID, ClassName, and Identify, don’t work.
XPath axes are used for dynamic content material the place regular locators will not work. Right here you find components based mostly on their relationship to different components. These are a number of the widespread XPath Axes locators;
Ancestor
The Ancestor Axis methodology is ideal for dealing with XML paperwork with extremely nested components. You possibly can choose all of the father or mother components, similar to grandparent and father or mother, of the present node from nearest to farthest.
We will have the next code;
<bookstore>
<e book>
<title>The Nice Gatsby</title>
<writer>F. Scott Fitzgerald</writer>
<style>Fiction</style>
</e book>
<e book>
<title>The Greatest Dilemma</title>
<writer>George Orwell</writer>
<style>Dystopian</style>
</e book>
</bookstore>
If we need to choose all of the ancestors of the ‘title’ aspect for the e book “The Greatest Dilemma”, we are able to use this Ancestor Axis methodology;
//title[text() = '1984']/ancestor::*
As a sequel to
The Following Axis methodology finds all nodes that place the present node’s closing tag. This methodology doesn’t take into account the hierarchy or location of the goal nodes. For instance, in case you have an XML doc or net web page with a number of sections, you’ll be able to determine a component that seems after a selected part with out having to navigate by the whole tree construction.
Older
The Dad or mum Axis methodology in XPath selects the father or mother of the present node. You need to use the next path to seek out the father or mother node;
//tag[@attribute='value']/father or mother::tagName
This strategy works when the kid components within the present node have distinctive attributes that you could simply discover and need to test with the father or mother node.
Baby
The Baby Axis methodology in XPath selects all youngsters of the present node. It stays some of the widespread XPath Axis strategies, because it helps choose baby nodes of a given aspect.
Take into account this little bit of code;
<part id='textual content'>
<p>Paragraph one</p>
<p>Paragraph two</p>
<p>Paragraph three</p>
<p>Paragraph 4</p>
</part>
We will find all ‘p’ components in our code utilizing this axis;
//part[@id='text']/baby::p
Regularly Requested Questions
CSS selectors can solely discover components based mostly on their ID, tag identify, and sophistication. Then again, you should use XPath to find components based mostly on their location, textual content content material, and different attributes within the HTML construction. You may as well retailer XPath expressions in variables and reuse them in your utility.
You need to use XPath with any language that Selenium helps. You possibly can write your scripts in JavaScript, Java, Python, Ruby, C# and PHP.
You need to use CSS selectors, picture recognition, or Selenium’s built-in locators as a substitute for XPath. CSS selectors are the most typical; you will discover components by their tag identify, ID or class. Picture recognition means that you can find components based mostly on their pictures. The Selenium’s built-in viewfinders are designed for simple use.
Conclusion
Now you can outline XPath in Selenium, differentiate between Absolute and Relative XPath, and find components utilizing totally different XPath locators. The selection of locator will depend on the character of the content material and your finish targets.
Take a look at our article on Selenium interview questions if you wish to be successful at your subsequent job interview.