Fashions and their relationships are the center of Laravel Eloquent. If they offer you a tough time otherwise you’re not capable of finding a easy, pleasant, and full information, begin right here!
Sitting on the opposite aspect of their programming article, it’s simple for the author to feign or blow up the aura of experience/status the platform offers. However I’ll be sincere — I had an extraordinarily laborious time studying Laravel, if solely as a result of it was my first full-stack framework. One motive was that I used to be not utilizing it at work and was exploring it out of curiosity; so, I’d make an try, get to some extent, get confused, surrender, and finally overlook every thing. I will need to have executed this 5-6 instances earlier than it began making sense to me (in fact, the documentation doesn’t assist).
However what nonetheless didn’t make sense was Eloquent. Or at the very least, the relationships between fashions (as a result of Eloquent is just too massive to study fully). Examples modeling authors and weblog posts are a joke as a result of actual tasks are way more complicated; sadly, the official docs use the exact same (or comparable) examples. Or even when I did come throughout some helpful article/useful resource, the reason was so unhealthy or so badly lacking that it was simply no use.
(By the way in which, I’ve been attacked for attacking the official documentation earlier than, so when you’re having comparable concepts, right here’s my customary reply: go take a look at the Django documentation after which speak to me.)
Finally, little by little, it did come collectively and made sense. I used to be lastly capable of mannequin tasks correctly and use the fashions comfortably. Then in the future I got here throughout some neat Collections methods that make this work extra nice. On this article, I intend to cowl all of it, ranging from the very fundamentals after which overlaying all doable use instances that you’ll encounter in actual tasks.
Why are Eloquent mannequin relationships laborious?
Sadly, I come throughout far too many Laravel builders who don’t perceive fashions correctly.
However why?
Even at this time, when there’s an explosion of programs, articles, and movies on Laravel, the general understanding is poor. I feel it’s an necessary level and is value some reflection.
In case you ask me, I’ll say that Eloquent mannequin relationships aren’t laborious in any respect. No less than when seen from the angle of the definition of “laborious”. Stay schema migrations are laborious; writing a brand new templating engine is difficult; contributing code to the core of Laravel is difficult. In comparison with these, studying and utilizing an ORM . . . effectively, that may’t be laborious! 🤭🤭
What really occurs is that PHP builders studying Laravel discover Eloquent laborious. That’s the actual underlying problem, and in my view, there are a number of components contributing to this (harsh, unpopular opinion alert!):
- Previous to Laravel, the publicity to a framework for many PHP builders has been CodeIgniter (it’s nonetheless alive, by the way in which, even when it’s develop into extra Laravel/CakePHP-like). Within the older CodeIgniter group (if there was one), the “finest apply” was to instantly stick SQL queries the place wanted. And although we’ve got a brand new CodeIgniter at this time, the habits have carried over. Consequently, when studying Laravel, the concept of an ORM is 100% new to PHP builders.
- Discarding the very small share of PHP uncovered to frameworks akin to Yii, CakePHP, and so on., the remaining are used to working in core PHP or in an surroundings akin to WordPress. And right here once more, an OOP-based mindset doesn’t exist, so a framework, a service container, a design sample, an ORM . . . these are alien ideas.
- There’s little to no idea of steady studying within the PHP world. The common developer is comfortable working with single-server setups utilizing relational databases and issuing queries written as strings. Asynchronous programming, net sockets, HTTP 2/3, Linux (overlook Docker), unit testing, Area-Pushed Design — these are all alien concepts to an amazing proportion of PHP builders. Consequently, studying up on one thing new and difficult, to the purpose that one finds it snug, doesn’t occur when Eloquent is encountered.
- The general understanding of databases and modeling is poor as effectively. Since database design is instantly, inseparably linked to Eloquent fashions, it raises the issue bar increased.
I don’t imply to be harsh and generalize globally — there are wonderful PHP builders as effectively, and lots of of them, however their total share may be very low.
In case you’re studying this, it means you’ve crossed all these limitations, come throughout Laravel, and messed with Eloquent.
Congratulations! 👏
You’re virtually there. All of the constructing blocks are in place and we simply must undergo them within the correct order and element. In different phrases, let’s begin on the database stage.
Database fashions: Relationships and Cardinality
To maintain issues easy, let’s assume we’re working with relational databases solely all through this text. One motive is that ORMs have been initially developed for relational databases; the opposite motive is that RDBMS are nonetheless overwhelmingly common.
Knowledge Mannequin
First, let’s perceive information fashions higher. The thought of a mannequin (or a information mannequin, to be extra exact), comes from the database. No database, no information, and so, no information mannequin. And what’s a knowledge mannequin? Fairly merely, it’s the way in which you determine to retailer/construction your information. For instance, in an e-commerce retailer, you may retailer every thing in a single big desk (HORRIBLE apply, however sadly, not unusual within the PHP world); that’d be your information mannequin. You may also cut up the info into 20 most important and 16 connecting tables; that’s a knowledge mannequin as effectively.
Additionally, word that the way in which information is structured within the database needn’t match 100% the way it’s organized within the framework’s ORM. Nonetheless, the trouble is at all times to maintain issues as shut as doable in order that we don’t have another factor to be conscious of when creating.
Cardinality
Let’s additionally get this time period out the way in which quick: cardinality. It simply refers to “rely”, loosely talking. So, 1, 2, 3 . . . can all be the cardinality of one thing. Finish of story. Let’s preserve shifting!
Relationships
Now, every time we retailer information in any kind of system, there are methods information factors might be associated to one another. I do know this sounds summary and boring, however bear with me somewhat. The methods totally different information objects are linked are often called relationships. Let’s see some non-database examples first in order that we’re satisfied we totally perceive the concept.
- If we retailer every thing in an array, one doable relationship is: the following information merchandise is at an index higher than the earlier index by
1
. - If we retailer information in a binary tree, one doable relationship is that the kid tree to the left at all times has smaller values than the mum or dad node’s (if we select to keep up the tree that manner).
- If we retailer information as an array of arrays of equal size, we are able to mimic a matrix, after which its properties develop into the relationships for our information.
So we see that the phrase “relationship”, within the context of information, doesn’t have a hard and fast that means. In truth, if two folks have been wanting on the similar information, they could determine two very totally different information relationships (whats up, statistics!) and each of them may very well be legitimate.
Relational databases
Based mostly on all of the phrases we’ve mentioned until now, we are able to lastly discuss one thing that has a direct hyperlink to fashions in an online framework (Laravel) — relational databases. For many of us, the first database used is MySQL, MariaDB, PostgreSQL, MSSQL, SQL Server, SQLite, or one thing alongside these strains. We additionally may vaguely know that these are referred to as RDBMS however most of us have forgotten what it really means and why does it matter.
The “R” in RDBMS stands for Relational, in fact. This isn’t an arbitrarily chosen time period; by this, we spotlight the truth that these database techniques are designed to work effectively with relationships between information saved. In truth, “relation” right here has a strict mathematical that means, and although no developer must trouble about it, it helps to know that there’s a rigorous mathematical basis beneath a majority of these databases.
Discover these sources to study SQL and NoSQL.
Okay, so we all know by expertise that information in RDBMS are saved as tables. The place, then, are the relationships?
Varieties of relationships in RDBMS
That is maybe crucial a part of your complete subject of Laravel and mannequin relationships. In case you don’t perceive this, Eloquent won’t ever make sense, so please concentrate for the following jiffy (it’s not even that troublesome).
An RDBMS permits us to have relationships between information — at a database stage. Because of this these relationships are usually not impractical/imaginary/subjective and might be created or inferred by totally different folks with the identical consequence.
On the similar time, there are particular capabilities/instruments inside an RDBMS that enable us to create and implement these relationships, akin to:
- Major Key
- Overseas Key
- Constraints
I don’t need this text to develop into a course in databases, so I’ll assume that you understand what these ideas are. If not, or in case you’re feeling shaky in your confidence, I like to recommend this pleasant video (be at liberty to discover your complete collection):
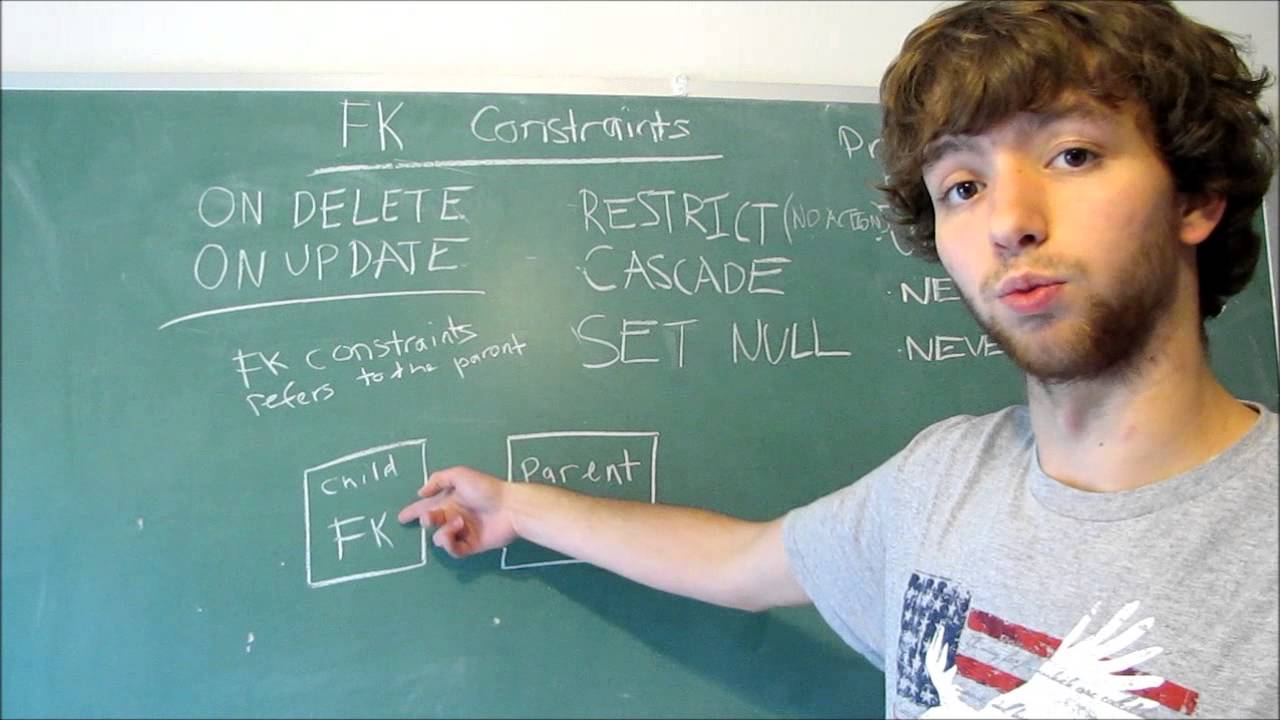
Because it occurs, these RDBMS-style relationships are additionally the commonest ones that happen in real-world purposes (not at all times, since a social community is finest modeled as a graph and never as a group of tables). So, let’s check out them one after the other and likewise attempt to perceive the place they is likely to be helpful.
One-to-one relationship
In virtually each net software, there are consumer accounts. Additionally, the next are true (typically talking) in regards to the customers and accounts:
- A consumer can have just one account.
- An account can solely be owned by one consumer.
Sure, we are able to argue that an individual can enroll with one other e mail and thus create two accounts, however from the angle of the online software, these are two totally different folks with two totally different accounts. The applying won’t, for instance, present one account’s information in one other.
What all this hair-splitting means is — if in case you have a state of affairs like this in your software and also you’re utilizing a relational database, you’d must design it as a one-to-one relationship. Notice that no one is forcing you artificially — there’s a transparent state of affairs within the enterprise area and you occur to be utilizing a relational database . . . solely when each these circumstances are glad, do you attain for a one-to-one relationship.
For this instance (customers and accounts), that is how we are able to implement this relationship when creating the schema:
CREATE TABLE customers(
id INT NOT NULL AUTO_INCREMENT,
e mail VARCHAR(100) NOT NULL,
password VARCHAR(100) NOT NULL,
PRIMARY KEY(id)
);
CREATE TABLE accounts(
id INT NOT NULL AUTO_INCREMENT,
position VARCHAR(50) NOT NULL,
PRIMARY KEY(id),
FOREIGN KEY(id) REFERENCES customers(id)
);
Discover the trick right here? It’s fairly unusual when constructing apps typically, however within the accounts
desk, we’ve got the sphere id
set as each major key and overseas key! The overseas key property hyperlinks it to the customers
desk (in fact 🙄) whereas the first key property makes the id
column distinctive — a real one-to-one relationship!
Granted, the constancy of this relationship shouldn’t be assured. As an example, there’s nothing stopping me from including 200 new customers with out including a single entry to the accounts
desk. If I try this, I find yourself with a one-to-zero relationship! 🤭🤭 However inside the bounds of pure construction, that’s one of the best we are able to do. If we need to forestall including customers with out accounts, we have to take assist from some form of programming logic, both within the type of database triggers or validations enforced by Laravel.
In case you’re starting to emphasize out, I’ve some superb recommendation:
- Take it sluggish. As sluggish as that you must. As an alternative of attempting to complete this text and the 15 others that you’ve got bookmarked for at this time, stick with this one. Let it take 3, 4, 5 days if that’s what it takes — your objective needs to be to knock Eloquent mannequin relationships off your record eternally. You’ve jumped from article to article earlier than, losing a number of hundred hours and but it didn’t assist. So, do one thing totally different this time. 😇
- Whereas this text is about Laravel Eloquent, all that comes a lot later. The inspiration of all of it is database schema, so our focus needs to be on getting that proper first. In case you can’t work purely on a database stage (assuming there are not any frameworks on the planet), then fashions and relationships won’t ever make full sense. So, overlook about Laravel for now. Fully. We’re solely speaking about and doing database design for now. Sure, I’ll make Laravel references at times, however your job is to disregard them fully in the event that they’re complicating the image for you.
- Afterward, learn somewhat extra on databases and what they provide. Indexes, efficiency, triggers, underlying information constructions and their habits, caching, relationships in MongoDB . . . no matter tangential matters you possibly can cowl will enable you as an engineer. Bear in mind, framework fashions are simply ghost shells; the actual performance of a platform comes from its underlying databases.
One-to-many relationship
I’m undecided when you realized this, however that is the kind of relationship all of us intuitively create in our on a regular basis work. After we create an orders
desk (a hypothetical instance), for instance, to retailer a overseas key to the customers
desk, we create a one-to-many relationship between customers and orders. Why is that? Nicely, have a look at it once more from the angle of who can have what number of: one consumer is allowed to have multiple order, which is just about how all e-commerce works. And seen from the other aspect, the connection says that an order can solely belong to 1 consumer, which additionally makes plenty of sense.
In information modeling, RDBMS books, and system documentation, this case is represented diagrammatically like this:
Discover the three strains making a trident of kinds? That is the image for “many”, and so this diagram says that one consumer can have many orders.
By the way in which, these “many” and “one” counts that we’re encountering repeatedly are what’s referred to as the Cardinality of a relationship (bear in mind this phrase from a earlier part?). Once more, for this text, the time period has no use, but it surely helps to know the idea in case it comes up throughout interviews or additional studying.
Easy, proper? And when it comes to precise SQL, creating this relationship can also be easy. In truth, it’s a lot easier than the case of a one-to-one relationship!
CREATE TABLE customers(
id INT NOT NULL AUTO_INCREMENT,
e mail VARCHAR(100) NOT NULL,
password VARCHAR(100) NOT NULL,
PRIMARY KEY(id)
);
CREATE TABLE orders(
id INT NOT NULL AUTO_INCREMENT,
user_id INT NOT NULL,
description VARCHAR(50) NOT NULL,
PRIMARY KEY(id),
FOREIGN KEY(user_id) REFERENCES customers(id)
);
The orders
desk shops consumer IDs for every order. Since there’s no constraint (restriction) that the consumer IDs within the orders
desk must be distinctive, it means we are able to repeat a single ID many instances. That is what creates the one-to-many relationship, and never some arcane magic that’s hidden beneath. The consumer IDs are saved form of in a dumb manner within the orders
desk, and SQL doesn’t have any idea of one-to-many, one-to-one, and so on. However as soon as we’re storing information this fashion, we are able to assume of there being a one-to-many relationship.
Hopefully, it’s making sense now. Or at the very least, extra sense than earlier than. 😅 Do not forget that similar to the rest, this can be a mere matter of apply, and when you’ve executed this 4-5 instances in real-world conditions, you’ll not even give it some thought.
Many-to-many relationships
The following kind of relationship that arises in apply is the so-called many-to-many relationship. As soon as once more, earlier than worrying about frameworks and even diving into databases, let’s consider a real-world analog: books and authors. Consider your favourite writer; they’ve written multiple guide, proper? On the similar time, it’s fairly frequent to see a number of authors collaborating on a guide (at the very least within the nonfiction style). So, one writer can write many books, and lots of authors can write one guide. Between the 2 entities (guide and writer), this varieties a many-to-many relationship.
Now, granted that you just’re extremely unlikely to create a real-world app involving libraries or books and authors, so let’s consider some extra examples. In a B2B setting, a producer orders objects from a provider and in flip receives an bill. The bill will include a number of line objects, every of them itemizing the amount and merchandise equipped; for instance, 5-inch pipe items x 200, and so on. On this state of affairs, objects and invoices have a many-to-many relationship (give it some thought and persuade your self). In a fleet administration system, automobiles and drivers can have an identical relationship. In an e-commerce website, customers and merchandise can have a many-to-many relationship if we contemplate performance akin to favorites or want lists.
Truthful sufficient, now methods to create this many-to-many relationship in SQL? Based mostly on our information of how the one-to-many relationship works, it is likely to be tempting to assume we should always retailer overseas keys to the opposite desk in each the tables. Nonetheless, we run into main issues if we attempt to do that. Take a look at this instance the place books are authors are speculated to have a many-to-many relationship:
At first look every thing seems to be all proper — books are mapped to authors precisely in a many-to-many style. However look carefully on the authors
desk information: guide ids 12 and 13 are each written by Peter M. (writer id 2), due to which we’ve got no alternative however to repeat the entries. Not solely does the authors
desk now have information integrity issues (correct normalization and all that), the values within the id
column at the moment are repeating. Because of this within the design we’ve chosen, there might be no major key column (as a result of major keys can’t have duplicate values), and every thing falls aside.
Clearly, we’d like a brand new manner to do that, and fortunately, this downside has already been solved. Since storing overseas keys instantly into each the tables screws issues up, The appropriate manner of making many-to-many relationships in RDBMS is by making a so-called “becoming a member of desk”. The thought is principally to let the 2 authentic tables stand undisturbed and create a 3rd desk to exhibit the many-to-many mapping.
Let’s redo the failed instance to include a becoming a member of desk:
Discover that there have been drastic modifications:
- The variety of columns within the
authors
desk is decreased. - The variety of columns within the
books
desk is decreased. - The variety of rows within the
authors
desk is decreased as there’s no want for repetition anymore. - A brand new desk referred to as
authors_books
has appeared, containing data about which writer id is linked to which guide id. We may’ve named the becoming a member of desk something, however by conference is the results of merely becoming a member of the 2 tables it represents, utilizing an underscore.
The becoming a member of desk has no major key and normally accommodates solely two columns — IDs from the 2 tables. It’s virtually as if we eliminated the overseas key columns from our earlier instance and pasted them into this new desk. Since there’s no major key, there might be as a lot repetition as is required to report all of the relationships.
Now, we are able to see with our eyes how the becoming a member of desk shows the relationships clearly, however how will we entry them in our purposes? The key is linked to the identify — becoming a member of desk. This isn’t a course on SQL queries so I received’t dive into it however the concept is that in order for you all of the books by a specific writer in a single, environment friendly question, you SQL-join the tables in the identical order –> authors
, authors_books
, and books
. The authors
and authors_books
tables are joined over the id
and author_id
columns, respectively, whereas the authors_books
and books
tables are joined on the book_id
and id
columns, respectively.
Exhausting, sure. However have a look at the intense aspect — we’ve completed all the required idea/groundwork we would have liked to do earlier than tackling Eloquent fashions. And let me remind you that each one these items shouldn’t be optionally available! Not figuring out database design will depart you in Eloquent confusion land eternally. Furthermore, no matter Eloquent does or tries to do, mirrors these database-level particulars completely, so it’s simple to see why attempting to study Eloquent whereas working away from RDBMS is an train in futility.
Creating mannequin relationships in Laravel Eloquent
Lastly, after a detour that lasted some 70,000 miles, we’ve reached the purpose the place we are able to discuss Eloquent, its fashions, and methods to create/use them. Now, we discovered within the earlier a part of the article that every thing begins with the database and the way you mannequin your information. This made me notice that I ought to use a single, full instance the place I begin a contemporary undertaking. On the similar time, I would like this instance to be real-world, and never about blogs and authors or books and cabinets (that are real-world, too, however have been executed to loss of life).
Let’s think about a retailer that sells tender toys. Let’s additionally assume that we’ve been offered the necessities doc, from which we are able to determine these 4 entities within the system: customers, orders, invoices, objects, classes, subcategories, and transactions. Sure, there’s prone to be extra complication concerned, however let’s simply put that apart and deal with how we go from a doc to an app.
As soon as the primary entities within the system have been recognized, we have to consider how they relate to one another, when it comes to the database relationships we’ve mentioned thus far. Listed below are those that I can consider:
- Customers and Orders: One to many.
- Orders and invoices: One to 1. I notice this one isn’t lower and dried, and relying on your online business area, there is likely to be a one to many, a many to 1, or a many to many relationship. However in the case of your common, small e-commerce retailer, one order will solely end in one bill and vice versa.
- Orders and Gadgets: Many to many.
- Gadgets and Classes: Many to 1. Once more, this isn’t so in massive e-commerce websites, however we’ve got a small operation.
- Classes and Subcategories: one to many. Once more, you’ll discover most real-world examples that contradict this, however hey, Eloquent is difficult sufficient as it’s, so let’s not make the info modeling tougher!
- Orders and Transactions: One to many. I’d additionally like so as to add these two factors as a justification for my alternative: 1) We may have added a relationship between Transactions and Invoices as effectively. It’s only a information modeling choice. 2) Why one to many right here? Nicely, it’s frequent that an order fee fails for some motive and succeeds the following time. On this case, we’ve got two transactions created for that order. Whether or not we want to present these failed transactions or not is a enterprise choice, but it surely’s at all times a good suggestion to seize helpful information.
Are there another relationships? Nicely, many extra relationships are doable, however they don’t seem to be sensible. For instance, we are able to say {that a} consumer has many transactions, so there needs to be a relationship between them. The factor to appreciate right here is that there’s already an oblique relationship: customers -> orders -> transactions, and customarily talking, it’s adequate as RDBMS are beasts in becoming a member of tables. Secondly, creating this relationship would imply including a user_id
column to the transactions
desk. If we did this for each doable direct relationship, then we’d be including much more load on the database (within the type of extra storage, particularly if UUIDs are getting used, and sustaining indexes), chaining down the general system. Certain, if the enterprise says they want transactions information and want it inside 1.5 seconds, we would determine so as to add that relationship and velocity issues up (tradeoffs, tradeoffs . . .).
And now, women and gents, the time has come to jot down the precise code!
Laravel mannequin relationships — actual instance with code
The following part of this text is about getting our arms soiled — however in a helpful manner. We’ll decide up the identical database entities as within the earlier e-commerce instance, and we’ll see how fashions in Laravel are created and linked, proper from putting in Laravel!
Naturally, I assume that you’ve got your improvement surroundings arrange and you know the way to put in and use Composer for managing dependencies.
$ composer international require laravel/installer -W
$ laravel new model-relationships-study
These two console instructions set up the Laravel installer (the -W
half is used for upgrading since I already had an older model put in). And in case you’re curious, as of writing, the Laravel model that acquired put in is 8.5.9. Do you have to panic and improve as effectively? I’d advise in opposition to it, since I don’t count on any main modifications between Laravel 5 and Laravel 8 within the context of our software. Some issues have modified and can affect this text (akin to Mannequin Factories), however I feel you’ll be capable to port the code.
Since we’ve already thought by way of the info mannequin and their relationships, the a part of creating the fashions shall be trivial. And also you’ll additionally see (I’m sounding like a damaged report now!) the way it mirrors the database schema because it’s 100% depending on it!
In different phrases, we have to first create the migrations (and mannequin recordsdata) for all of the fashions, which shall be utilized to the database. Later, we are able to work on the fashions and tack on the relationships.
So, which mannequin do we start with? The only and the least linked one, in fact. In our case, this implies the Person
mannequin. Since Laravel ships with this mannequin (and can’t work with out it 🤣), let’s modify the migration file and likewise clear up the mannequin to swimsuit our easy wants.
Right here’s the migration class:
class CreateUsersTable extends Migration
{
public perform up()
{
Schema::create('customers', perform (Blueprint $desk) {
$table->id();
$table->string('identify');
});
}
}
Since we’re not really constructing a undertaking, we don’t must get into passwords, is_active, and all that. Our customers
desk can have solely two columns: the id and the identify of the consumer.
Let’s create the migration for Class
subsequent. Since Laravel permits us the comfort to generate the mannequin too in a single command, we’ll reap the benefits of that, although we received’t contact the mannequin file for now.
$ php artisan make:mannequin Class -m
Mannequin created efficiently.
Created Migration: 2021_01_26_093326_create_categories_table
And right here’s the migration class:
class CreateCategoriesTable extends Migration
{
public perform up()
{
Schema::create('classes', perform (Blueprint $desk) {
$table->id();
$table->string('identify');
});
}
}
In case you’re shocked on the absence of the down()
perform, don’t be; in apply, you hardly ever find yourself utilizing it as dropping a column or desk or altering a column kind leads to information loss that may’t be recovered. In improvement, you’ll end up dropping your complete database after which re-running the migrations. However we digress, so let’s get again and sort out the following entity. Since subcategories are instantly associated to classes, I feel it’s a good suggestion to do this subsequent.
$ php artisan make:mannequin SubCategory -m
Mannequin created efficiently.
Created Migration: 2021_01_26_140845_create_sub_categories_table
All proper, now let’s replenish the migration file:
class CreateSubCategoriesTable extends Migration
{
public perform up()
{
Schema::create('sub_categories', perform (Blueprint $desk) {
$table->id();
$table->string('identify');
$table->unsignedBigInteger('category_id');
$table->overseas('category_id')
->references('id')
->on('classes')
->onDelete('cascade');
});
}
}
As you possibly can see, we add a separate column right here, referred to as category_id
, which is able to retailer IDs from the classes
desk. No prizes for guessing, this creates a one to many relationships on the database stage.
Now it’s the flip for objects:
$ php artisan make:mannequin Merchandise -m
Mannequin created efficiently.
Created Migration: 2021_01_26_141421_create_items_table
And the migration:
class CreateItemsTable extends Migration
{
public perform up()
{
Schema::create('objects', perform (Blueprint $desk) {
$table->id();
$table->string('identify');
$table->textual content('description');
$table->string('kind');
$table->unsignedInteger('value');
$table->unsignedInteger('quantity_in_stock');
$table->unsignedBigInteger('sub_category_id');
$table->overseas('sub_category_id')
->references('id')
->on('sub_categories')
->onDelete('cascade');
});
}
}
In case you really feel like issues needs to be executed otherwise, that’s nice. Two folks will hardly ever give you the very same schema and structure. Do word one factor, which is a finest apply of kinds: I’ve saved the priced as an integer.
Why?
Nicely, people realized that dealing with float divisions and all was ugly and error-prone on the database aspect, in order that they began saved the worth when it comes to the smallest forex unit. For instance, if we have been working in USD, the worth subject right here would characterize cents. All through the system the values and calculations shall be in cents; solely when it’s time to show to the consumer or ship a PDF by e mail, will we divide by 100 and spherical off. Intelligent, eh?
Anyway, discover that an merchandise is linked to a subcategory in a many-to-one relationship. It’s additionally linked to a class . . . not directly by way of its subcategory. We’ll see stable demonstrations of all these gymnastics, however for now, we have to admire the ideas and ensure we’re 100% clear.
Subsequent up is the Order
mannequin and its migration:
$ php artisan make:mannequin Order -m
Mannequin created efficiently.
Created Migration: 2021_01_26_144157_create_orders_table
For the sake of brevity, I’ll embody solely among the necessary fields within the migration. By that I imply, an order’s particulars can include a terrific many issues, however we’ll prohibit them to some in order that we are able to deal with the idea of mannequin relationships.
class CreateOrdersTable extends Migration
{
public perform up()
{
Schema::create('orders', perform (Blueprint $desk) {
$table->id();
$table->string('standing');
$table->unsignedInteger('total_value');
$table->unsignedInteger('taxes');
$table->unsignedInteger('shipping_charges');
$table->unsignedBigInteger('user_id');
$table->overseas('user_id')
->references('id')
->on('customers')
->onDelete('cascade');
});
}
}
Appears nice, however, wait a minute! The place are the objects on this order? As we established earlier, there’s a many-to-many relationship between orders and objects, so a easy overseas key doesn’t work. The answer is a so-called becoming a member of desk or intermediate desk. In different phrases, we’d like a becoming a member of desk to retailer the many-to-many mapping between orders and objects. Now, within the Laravel world, there’s a built-in conference that we comply with to avoid wasting time: If I create a brand new desk through the use of the singular type of the 2 desk names, place them in dictionary order, and be part of them utilizing an underscore, Laravel will mechanically acknowledge it because the becoming a member of desk.
In our case, the becoming a member of desk shall be referred to as item_order
(the phrase “merchandise” comes earlier than “order” in a dictionary). Additionally, as defined earlier than, this becoming a member of desk will usually include solely two columns, overseas keys to every desk.
We may create a mannequin + migration right here, however the mannequin won’t ever be used because it’s extra of a meta factor. Thus, we create a brand new migration in Laravel and inform it what’s what.
$ php artisan make:migration create_item_order_table --create="item_order"
Created Migration: 2021_01_27_093127_create_item_order_table
This leads to a brand new migration, which we are going to change as follows:
class CreateItemOrderTable extends Migration
{
public perform up()
{
Schema::create('item_order', perform (Blueprint $desk) {
$table->unsignedBigInteger('order_id');
$table->overseas('order_id')
->references('id')
->on('orders')
->onDelete('cascade');
$table->unsignedBigInteger('item_id');
$table->overseas('item_id')
->references('id')
->on('objects')
->onDelete('cascade');
});
}
}
The right way to really entry these relationships by way of Eloquent technique calls is a subject for later, however discover that we have to first painstakingly, by hand, create these overseas keys. With out these, there’s no Eloquent and there’s no “sensible” in Laravel. 🙂
Are we there but? Nicely, virtually . . .
We solely have a pair extra fashions to fret about. The primary one is the invoices desk, and also you’ll keep in mind that we determined to make it a one-to-one relationship with orders.
$ php artisan make:mannequin Bill -m
Mannequin created efficiently.
Created Migration: 2021_01_27_101116_create_invoices_table
Within the very early sections of this text, we noticed that one approach to implement a one-to-one relationship is to make the first key on the kid desk the overseas key as effectively. In apply, hardly anybody takes this overly cautious method, and other people typically design the schema as they might for a one-to-many relationship. My take is {that a} center method is healthier; simply make the overseas key distinctive and also you’ve made positive that the mum or dad mannequin’s IDs can’t be repeated:
class CreateInvoicesTable extends Migration
{
public perform up()
{
Schema::create('invoices', perform (Blueprint $desk) {
$table->id();
$table->timestamp('raised_at')->nullable();
$table->string('standing');
$table->unsignedInteger('totalAmount');
$table->unsignedBigInteger('order_id')->distinctive();
$table->overseas('order_id')
->references('id')
->on('orders')
->onDelete('cascade')
->distinctive();
});
}
}
And sure, for the umpteenth time, I’m conscious that this invoices desk has so much lacking; nevertheless, our focus right here is to see how mannequin relationships work and to not design a whole database.
Okay, so, we’ve reached the purpose the place we have to create the ultimate migration of our system (I hope!). The main focus is now on the Transaction
mannequin, which we determined earlier is linked to the Order
mannequin. By the way in which, right here’s an train for you: Ought to the Transaction
mannequin be as an alternative linked to the Bill
mannequin? Why and why not? 🙂
$ php artisan make:mannequin Transaction -m
Mannequin created efficiently.
Created Migration: 2021_01_31_145806_create_transactions_table
And the migration:
class CreateTransactionsTable extends Migration
{
public perform up()
{
Schema::create('transactions', perform (Blueprint $desk) {
$table->id();
$table->timestamp('executed_at');
$table->string('standing');
$table->string('payment_mode');
$table->string('transaction_reference')->nullable();
$table->unsignedBigInteger('order_id');
$table->overseas('order_id')
->references('id')
->on('orders')
->onDelete('cascade');
});
}
}
Phew! That was some laborious work . . . let’s run the migrations and see how we’re doing within the eyes of the database.
$ php artisan migrate:contemporary
Dropped all tables efficiently.
Migration desk created efficiently.
Migrating: 2014_10_12_000000_create_users_table
Migrated: 2014_10_12_000000_create_users_table (3.45ms)
Migrating: 2021_01_26_093326_create_categories_table
Migrated: 2021_01_26_093326_create_categories_table (2.67ms)
Migrating: 2021_01_26_140845_create_sub_categories_table
Migrated: 2021_01_26_140845_create_sub_categories_table (3.83ms)
Migrating: 2021_01_26_141421_create_items_table
Migrated: 2021_01_26_141421_create_items_table (6.09ms)
Migrating: 2021_01_26_144157_create_orders_table
Migrated: 2021_01_26_144157_create_orders_table (4.60ms)
Migrating: 2021_01_27_093127_create_item_order_table
Migrated: 2021_01_27_093127_create_item_order_table (3.05ms)
Migrating: 2021_01_27_101116_create_invoices_table
Migrated: 2021_01_27_101116_create_invoices_table (3.95ms)
Migrating: 2021_01_31_145806_create_transactions_table
Migrated: 2021_01_31_145806_create_transactions_table (3.54ms)
Reward be to the Lord! 🙏🏻🙏🏻 Appears like we’ve survived the second of trial.
And with that, we’re prepared to maneuver on to defining mannequin relationships! For that, we have to return to the record we created earlier, outlining the kind of direct relationships between fashions (tables).
To start with, we’ve established that there’s a one-to-many relationship between customers and orders. We are able to verify this by going to the orders’ migration file and seeing the presence of the sphere user_id
there. This subject is what creates the connection, as a result of any relationship we’re fascinated about establishing must be honored by the database first; the remaining (Eloquent syntax and the place to jot down which perform) is simply pure formality.
In different phrases, the connection is already there. We simply want to inform Eloquent to make it accessible at run time. Let’s begin with the Order
mannequin, the place we declare that it belongs to the Person
mannequin:
<?php
namespace AppModels;
use IlluminateDatabaseEloquentFactoriesHasFactory;
use IlluminateDatabaseEloquentModel;
class Order extends Mannequin
{
use HasFactory;
public perform consumer() {
return $this->belongsTo(Person::class);
}
}
The syntax have to be acquainted to you; we declare a perform named consumer()
, which serves to entry the consumer that owns this order (the perform identify might be something; it’s what it returns that issues). Assume again once more for a second — if there was no database and no overseas keys, an announcement like $this->belongsTo
can be meaningless. It’s solely as a result of there’s a overseas key on the orders
desk that Laravel is ready to use that user_id
to search for the consumer with similar id
and return it. By itself, with out the cooperation of the database, Laravel can not create relationships out of skinny air.
Now, it’d even be good to have the ability to write $user->orders
to entry a consumer’s orders. This implies we have to go to the Person
mannequin and write out a perform for the “many” a part of this one-to-many relationship:
<?php
namespace AppModels;
use IlluminateDatabaseEloquentFactoriesHasFactory;
use IlluminateDatabaseEloquentModel;
class Person extends Mannequin
{
use HasFactory;
public $timestamps = false;
public perform orders() {
return $this->hasMany(Order::class);
}
}
Sure, I closely modified the default Person
mannequin as a result of we don’t want all the opposite performance for this tutorial. Anyway, the Person
class now has a way referred to as orders()
, which says that one consumer might be related to a number of orders. Within the ORM world, we are saying that the orders()
relationship right here is the inverse of the consumer()
relationship we had on the Order
mannequin.
However, wait a minute! How is that this relationship working? I imply, there’s nothing on the database stage that has a number of connections going out from the customers
desk to the orders
desk.
Truly, there is an present connection, and seems, it’s sufficient by itself — the overseas key reference saved within the orders
desk! That is, once we say one thing like $user->orders
, Laravel hits the orders()
perform and is aware of by taking a look at it that there’s a overseas key on the orders
desk. Then, it kinda does a SELECT * FROM orders WHERE user_id = 23
and returns the question outcomes as a group. After all, the entire level of getting an ORM is to overlook about SQL, however we shouldn’t fully overlook that the underlying base is the RDBMS that runs SQL queries.
Subsequent, let’s breeze by way of the orders and invoices fashions, the place we’ve got a one-to-one relationship:
<?php
namespace AppModels;
use IlluminateDatabaseEloquentFactoriesHasFactory;
use IlluminateDatabaseEloquentModel;
class Order extends Mannequin
{
use HasFactory;
public $timestamps = false;
public perform consumer() {
return $this->belongsTo(Person::class);
}
public perform bill() {
return $this->hasOne(Bill::class);
}
}
And the bill mannequin:
<?php
namespace AppModels;
use IlluminateDatabaseEloquentFactoriesHasFactory;
use IlluminateDatabaseEloquentModel;
class Bill extends Mannequin
{
use HasFactory;
public $timestamps = false;
public perform order() {
return $this->belongsTo(Order::class);
}
}
Discover that on the database stage, in addition to virtually on the Eloquent stage, it’s a typical one-to-many relationship; we’ve simply added some checks to verify it stays one-to-one.
We now come to a different kind of relationship — the many-to-many between orders and objects. Recall that we have already got created an intermediate desk referred to as item_order
that shops the mapping between the first keys. If this a lot has been executed appropriately, defining the connection and dealing with it’s trivial. As per the Laravel docs, to outline a many-to-many relationship, your strategies should return a belongsToMany()
occasion.
So, within the Merchandise
mannequin:
<?php
namespace AppModels;
use IlluminateDatabaseEloquentFactoriesHasFactory;
use IlluminateDatabaseEloquentModel;
class Merchandise extends Mannequin
{
use HasFactory;
public $timestamps = false;
public perform orders() {
return $this->belongsToMany(Order::class);
}
}
Surprisingly, the inverse relationship is sort of an identical:
class Order extends Mannequin
{
/* ... different code */
public perform objects() {
return $this->belongsToMany(Merchandise::class);
}
}
And that’s it! So long as we’ve adopted the naming conventions appropriately, Laravel is ready to deduce the mappings in addition to their location.
Since all of the three basic varieties of relationships have been coated (one-to-one, one-to-many, many-to-many), I’ll cease writing out the strategies for different fashions, as they’ll be alongside the identical strains. As an alternative, let’s create the factories for these fashions, create some dummy information, and see these relationships in motion!
How will we try this? Nicely, let’s take the quick-and-dirty path and throw every thing into the default seeder file. Then, once we run the migrations, we are going to run the seeder as effectively. So, right here’s what my DatabaseSeeder.php
file seems to be like:
<?php
namespace DatabaseSeeders;
use IlluminateDatabaseSeeder;
use AppModelsCategory;
use AppModelsSubCategory;
use AppModelsItem;
use AppModelsOrder;
use AppModelsInvoice;
use AppModelsUser;
use Faker;
class DatabaseSeeder extends Seeder
{
public perform run()
{
$faker = FakerFactory::create();
// Let's make two customers
$user1 = Person::create(['name' => $faker->name]);
$user2 = Person::create(['name' => $faker->name]);
// Create two classes, every having two subcategories
$category1 = Class::create(['name' => $faker->word]);
$category2 = Class::create(['name' => $faker->word]);
$subCategory1 = SubCategory::create(['name' => $faker->word, 'category_id' => $category1->id]);
$subCategory2 = SubCategory::create(['name' => $faker->word, 'category_id' => $category1->id]);
$subCategory3 = SubCategory::create(['name' => $faker->word, 'category_id' => $category2->id]);
$subCategory4 = SubCategory::create(['name' => $faker->word, 'category_id' => $category2->id]);
// After classes, effectively, we've got objects
// Let's create two objects every for sub-category 2 and 4
$item1 = Merchandise::create([
'sub_category_id' => 2,
'name' => $faker->name,
'description' => $faker->text,
'type' => $faker->word,
'price' => $faker->randomNumber(2),
'quantity_in_stock' => $faker->randomNumber(2),
]);
$item2 = Merchandise::create([
'sub_category_id' => 2,
'name' => $faker->name,
'description' => $faker->text,
'type' => $faker->word,
'price' => $faker->randomNumber(3),
'quantity_in_stock' => $faker->randomNumber(2),
]);
$item3 = Merchandise::create([
'sub_category_id' => 4,
'name' => $faker->name,
'description' => $faker->text,
'type' => $faker->word,
'price' => $faker->randomNumber(4),
'quantity_in_stock' => $faker->randomNumber(2),
]);
$item4 = Merchandise::create([
'sub_category_id' => 4,
'name' => $faker->name,
'description' => $faker->text,
'type' => $faker->word,
'price' => $faker->randomNumber(1),
'quantity_in_stock' => $faker->randomNumber(3),
]);
// Now that we've got customers and objects, let's make user1 place a few orders
$order1 = Order::create([
'status' => 'confirmed',
'total_value' => $faker->randomNumber(3),
'taxes' => $faker->randomNumber(1),
'shipping_charges' => $faker->randomNumber(2),
'user_id' => $user1->id
]);
$order2 = Order::create([
'status' => 'waiting',
'total_value' => $faker->randomNumber(3),
'taxes' => $faker->randomNumber(1),
'shipping_charges' => $faker->randomNumber(2),
'user_id' => $user1->id
]);
// now, assigning objects to orders
$order1->objects()->connect($item1);
$order1->objects()->connect($item2);
$order1->objects()->connect($item3);
$order2->objects()->connect($item1);
$order2->objects()->connect($item4);
// and at last, create invoices
$invoice1 = Bill::create([
'raised_at' => $faker->dateTimeThisMonth(),
'status' => 'settled',
'totalAmount' => $faker->randomNumber(3),
'order_id' => $order1->id,
]);
}
}
And now we arrange the database once more and seed it:
$ php artisan migrate:contemporary --seed
Dropped all tables efficiently.
Migration desk created efficiently.
Migrating: 2014_10_12_000000_create_users_table
Migrated: 2014_10_12_000000_create_users_table (43.81ms)
Migrating: 2021_01_26_093326_create_categories_table
Migrated: 2021_01_26_093326_create_categories_table (2.20ms)
Migrating: 2021_01_26_140845_create_sub_categories_table
Migrated: 2021_01_26_140845_create_sub_categories_table (4.56ms)
Migrating: 2021_01_26_141421_create_items_table
Migrated: 2021_01_26_141421_create_items_table (5.79ms)
Migrating: 2021_01_26_144157_create_orders_table
Migrated: 2021_01_26_144157_create_orders_table (6.40ms)
Migrating: 2021_01_27_093127_create_item_order_table
Migrated: 2021_01_27_093127_create_item_order_table (4.66ms)
Migrating: 2021_01_27_101116_create_invoices_table
Migrated: 2021_01_27_101116_create_invoices_table (6.70ms)
Migrating: 2021_01_31_145806_create_transactions_table
Migrated: 2021_01_31_145806_create_transactions_table (6.09ms)
Database seeding accomplished efficiently.
All, proper! Now could be the ultimate a part of this text, the place we merely entry these relationships and make sure all that we’ve discovered thus far. You’ll be delighted to know (I hope) that this shall be a light-weight and enjoyable part.
And now, let’s fireplace up probably the most enjoyable Laravel element — the Tinker interactive console!
$ php artisan tinker
Psy Shell v0.10.6 (PHP 8.0.0 — cli) by Justin Hileman
>>>
Accessing one-to-one mannequin relationships in Laravel Eloquent
Okay, so, first, let’s entry the one-to-one relationship we’ve got in our fashions of order and bill:
>>> $order = Order::discover(1);
[!] Aliasing 'Order' to 'AppModelsOrder' for this Tinker session.
=> AppModelsOrder {#4108
id: 1,
standing: "confirmed",
total_value: 320,
taxes: 5,
shipping_charges: 12,
user_id: 1,
}
>>> $order->bill
=> AppModelsInvoice {#4004
id: 1,
raised_at: "2021-01-21 19:20:31",
standing: "settled",
totalAmount: 314,
order_id: 1,
}
Discover one thing? Do not forget that the way in which it’s been executed on the database-level, this relationship is a one-to-many, if not for the additional constraints. So, Laravel may’ve returned a group of objects (or just one object) because the consequence, and that will be technically correct. BUT . . . we’ve advised Laravel that it’s a one-to-one relationship, so, the result’s a single Eloquent occasion. Discover how the identical factor occurs when accessing the inverse relationship:
$bill = Bill::discover(1);
[!] Aliasing 'Bill' to 'AppModelsInvoice' for this Tinker session.
=> AppModelsInvoice {#3319
id: 1,
raised_at: "2021-01-21 19:20:31",
standing: "settled",
totalAmount: 314,
order_id: 1,
}
>>> $invoice->order
=> AppModelsOrder {#4042
id: 1,
standing: "confirmed",
total_value: 320,
taxes: 5,
shipping_charges: 12,
user_id: 1,
}
Accessing one-to-many mannequin relationships in Laravel Eloquent
We now have a one-to-many relationship between customers and orders. Let’s “tinker” with it now and see the output:
>>> Person::discover(1)->orders;
[!] Aliasing 'Person' to 'AppModelsUser' for this Tinker session.
=> IlluminateDatabaseEloquentCollection {#4291
all: [
AppModelsOrder {#4284
id: 1,
status: "confirmed",
total_value: 320,
taxes: 5,
shipping_charges: 12,
user_id: 1,
},
AppModelsOrder {#4280
id: 2,
status: "waiting",
total_value: 713,
taxes: 4,
shipping_charges: 80,
user_id: 1,
},
],
}
>>> Order::discover(1)->consumer
=> AppModelsUser {#4281
id: 1,
identify: "Dallas Kshlerin",
}
Precisely as anticipated, accessing a consumer’s orders leads to a group of data, whereas the inverse produces only one single Person
object. In different phrases, one-to-many.
Accessing many-to-many mannequin relationships in Laravel Eloquent
Now, let’s discover a relationship that’s many-to-many. We now have one such relationship between objects and orders:
>>> $item1 = Merchandise::discover(1);
[!] Aliasing 'Merchandise' to 'AppModelsItem' for this Tinker session.
=> AppModelsItem {#4253
id: 1,
identify: "Russ Kutch",
description: "Deserunt voluptatibus omnis ut cupiditate doloremque. Perspiciatis officiis odio et accusantium alias aut. Voluptatum provident aut ut et.",
kind: "adipisci",
value: 26,
quantity_in_stock: 65,
sub_category_id: 2,
}
>>> $order1 = Order::discover(1);
=> AppModelsOrder {#4198
id: 1,
standing: "confirmed",
total_value: 320,
taxes: 5,
shipping_charges: 12,
user_id: 1,
}
>>> $order1->objects
=> IlluminateDatabaseEloquentCollection {#4255
all: [
AppModelsItem {#3636
id: 1,
name: "Russ Kutch",
description: "Deserunt voluptatibus omnis ut cupiditate doloremque. Perspiciatis officiis odio et accusantium alias aut. Voluptatum provident aut ut et.",
type: "adipisci",
price: 26,
quantity_in_stock: 65,
sub_category_id: 2,
pivot: IlluminateDatabaseEloquentRelationsPivot {#4264
order_id: 1,
item_id: 1,
},
},
AppModelsItem {#3313
id: 2,
name: "Mr. Green Cole",
description: "Maxime beatae porro commodi fugit hic. Et excepturi natus distinctio qui sit qui. Est non non aut necessitatibus aspernatur et aspernatur et. Voluptatem possimus consequatur exercitationem et.",
type: "pariatur",
price: 381,
quantity_in_stock: 82,
sub_category_id: 2,
pivot: IlluminateDatabaseEloquentRelationsPivot {#4260
order_id: 1,
item_id: 2,
},
},
AppModelsItem {#4265
id: 3,
name: "Brianne Weissnat IV",
description: "Delectus ducimus quia voluptas fuga sed eos esse. Rerum repudiandae incidunt laboriosam. Ea eius omnis autem. Cum pariatur aut voluptas sint aliquam.",
type: "non",
price: 3843,
quantity_in_stock: 26,
sub_category_id: 4,
pivot: IlluminateDatabaseEloquentRelationsPivot {#4261
order_id: 1,
item_id: 3,
},
},
],
}
>>> $item1->orders
=> IlluminateDatabaseEloquentCollection {#4197
all: [
AppModelsOrder {#4272
id: 1,
status: "confirmed",
total_value: 320,
taxes: 5,
shipping_charges: 12,
user_id: 1,
pivot: IlluminateDatabaseEloquentRelationsPivot {#4043
item_id: 1,
order_id: 1,
},
},
AppModelsOrder {#4274
id: 2,
status: "waiting",
total_value: 713,
taxes: 4,
shipping_charges: 80,
user_id: 1,
pivot: IlluminateDatabaseEloquentRelationsPivot {#4257
item_id: 1,
order_id: 2,
},
},
],
}
This output could be a bit dizzying to learn by way of however discover that item1 is a part of order1’s objects, and vice versa, which is how we set issues up. Let’s additionally peek into the intermediate desk that shops the mappings:
>>> use DB;
>>> DB::desk('item_order')->choose('*')->get();
=> IlluminateSupportCollection {#4290
all: [
{#4270
+"order_id": 1,
+"item_id": 1,
},
{#4276
+"order_id": 1,
+"item_id": 2,
},
{#4268
+"order_id": 1,
+"item_id": 3,
},
{#4254
+"order_id": 2,
+"item_id": 1,
},
{#4267
+"order_id": 2,
+"item_id": 4,
},
],
}
Conclusion
Sure, that is it, actually! It’s been a very lengthy article, however I hope it has been helpful. Is that each one one must find out about Laravel fashions?
Sadly, no. The rabbit gap is basically, actually deep, and there are lots of tougher ideas akin to Polymorphic Relationships and efficiency tuning, and whatnot, which you’ll encounter as you develop as a Laravel developer. For now, what this text covers is sufficient for 70% of the builders 70% of the time, roughly talking. It is going to be actually lengthy earlier than you’ll really feel the necessity to improve your information.
With that caveat out of the way in which, I would like you to remove this most necessary perception: nothing is darkish magic or out of attain in programming. It’s solely that we don’t perceive the foundations and the way issues are constructed, which makes us wrestle and really feel pissed off.
So . . . ?
Spend money on your self! Programs, books, articles, different programming communities (Python is my #1 advice) — use no matter sources you will discover and devour them often if slowly. Fairly quickly, the variety of situations the place you’re prone to botch the entire thing will diminish drastically.
Okay, sufficient preaching. Have a pleasant day! 🙂