On this tutorial you’ll discover ways to use the timeit perform from Python’s timeit module. You’ll discover ways to time easy expressions and features in Python.
Timing your code permits you to get an estimate of the execution time of a bit of code and in addition helps you determine the elements of the code that must be optimized.
We’ll begin by studying Python syntax timeit
perform. And after that, we are going to present code examples to grasp how you can use it to time blocks of code and features in your Python module. Let’s begin.
The right way to use the Python timeit perform
The timeit
module is a part of Python’s commonplace library and you’ll import it:
import timeit
The syntax for the timeit
perform from the timeit
module is as proven beneath:
timeit.timeit(stmt, setup, quantity)
Right here:
stmt
is the piece of code whose execution time is to be measured. You may specify it as a easy Python string or multiline string, or cross the identify of the queryable string.- Because the identify suggests,
setup
denotes the piece of code that must be executed solely as soon as, usually as a situation for itstmt
run. For instance, suppose you calculate the execution time to create a NumPy array. On this case importnumpy
is thesetup
code and the precise creation is the instruction to be timed. - The parameters
quantity
signifies the variety of occasions thestmt
is carried out. The default worth ofquantity
is 1 million (1000000), however you can even set this parameter to any worth of your alternative.
Now that we have realized the syntax to make use of the timeit()
perform, let’s begin by coding some examples.
Timing of easy Python expressions
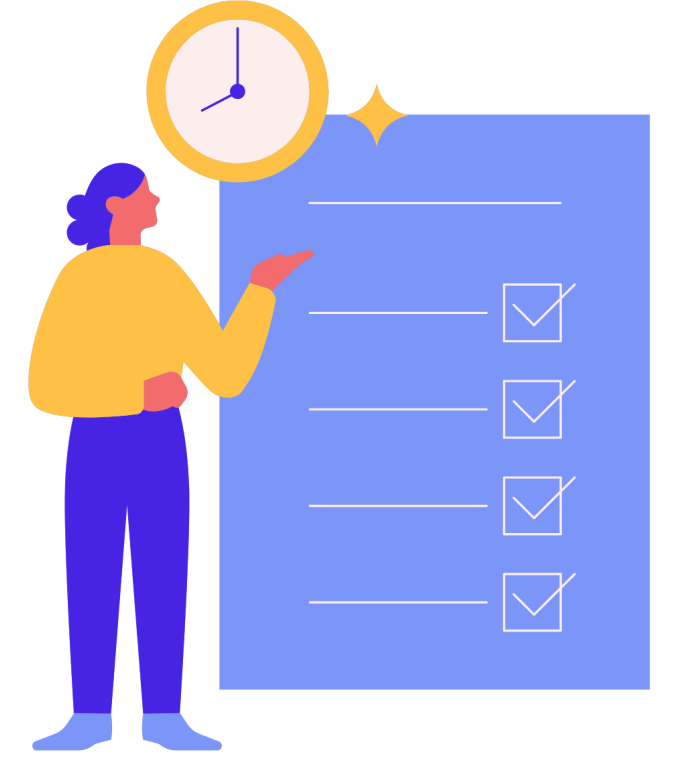
On this part we attempt to measure the execution time of easy Python expressions utilizing timeit.
Begin a Python REPL and run the next code samples. Right here we calculate the execution time of exponentiation and deepening operations for 10,000 and 100,000 runs.
Be aware that we cross the assertion to be timed as a Python string and use a semicolon to separate the totally different expressions within the assertion.
>>> import timeit
>>> timeit.timeit('3**4;3//4',quantity=10000)
0.0004020999999738706
>>> timeit.timeit('3**4;3//4',quantity=100000)
0.0013780000000451764
Run Python timeit on the command line
You can even use timeit
on the command line. Right here is the command line equal of the timeit perform name:
$ python-m timeit -n [number] -s [setup] [stmt]
python -m timeit
represents that we runtimeit
as fundamental module.n
is a command line choice that specifies the variety of occasions to execute the code. That is equal to thequantity
argument within thetimeit()
perform name.- You should utilize the choice
-s
to outline the setup code.
Right here we rewrite the earlier instance utilizing the command line equal:
$ python -m timeit -n 100000 '3**4;3//4'
100000 loops, better of 5: 35.8 nsec per loop
On this instance, we calculate the execution time of the built-in file len()
perform. The initialization of the string is the set up code handed when utilizing the s
alternative.
$ python -m timeit -n 100000 -s "string_1 = 'coding'" 'len(string_1)'
100000 loops, better of 5: 239 nsec per loop
Discover within the output that we get the execution time for better of 5 runs. What does this imply? While you run timeit
on the command line, the repeat
alternative r
is about to the commonplace worth of 5. This implies the execution of the stmt
for the desired quantity
variety of occasions iterates 5 occasions and returns the perfect execution occasions.
Evaluation of string reversal strategies utilizing timeit
Should you’re working with Python strings, it’s possible you’ll wish to reverse them. The 2 most typical approaches to reversing strings are as follows:
- Utilizing string slicing
- The habits
reversed()
perform and thebe part of()
methodology
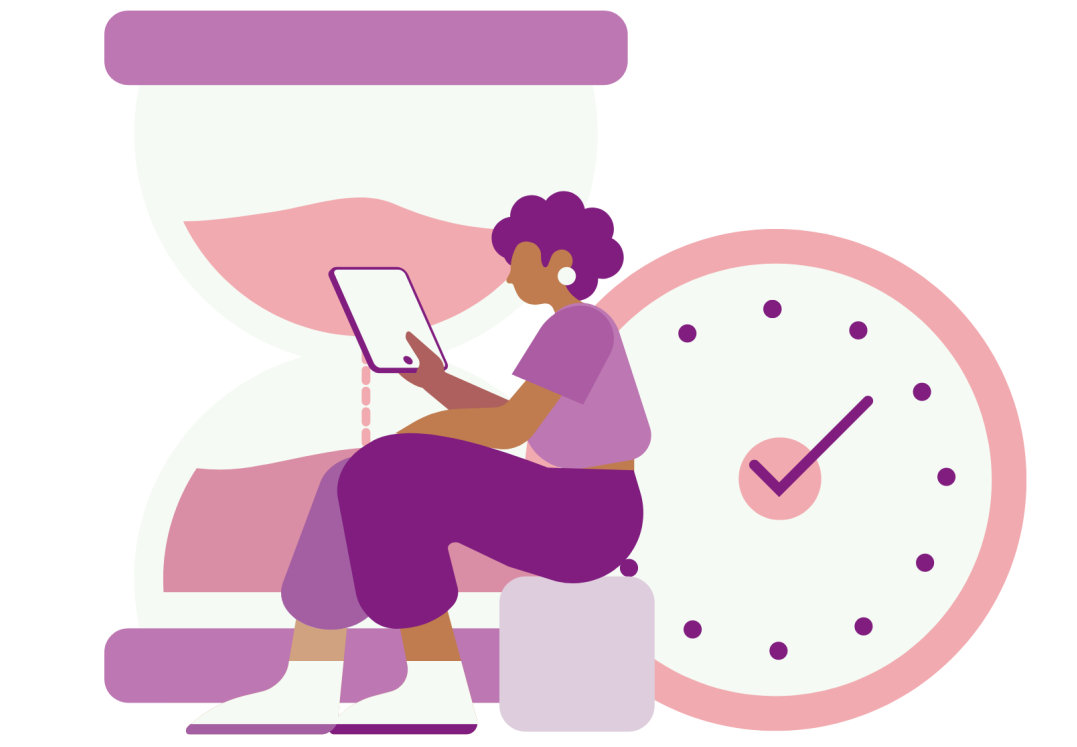
Reverse Python strings utilizing string slicing
Let’s have a look at how string slicing works, and the way you should utilize it to reverse a Python string. Utilizing the syntax some-string[start:stop]
returns a part of the string, beginning on the index begin
and extends to the index stop-1
. Let’s take an instance.
Contemplate the next string ‘Python’. The string has a size of 6 and the listing of indices is 0, 1, 2 via 5.
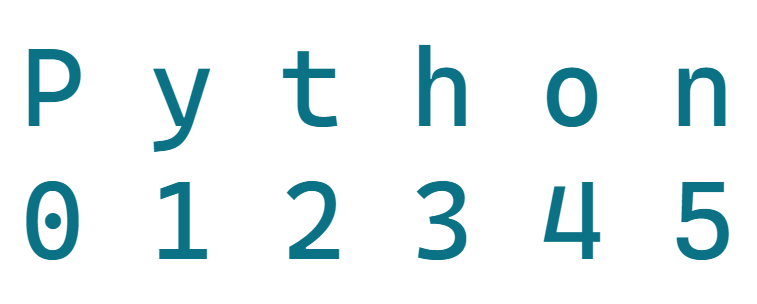
>>> string_1 = 'Python'
While you use each the begin
and the cease
values, you get a string phase that extends from begin
Disagreeable stop-1
. Due to this fact, string_1[1:4]
returns ‘yth’.
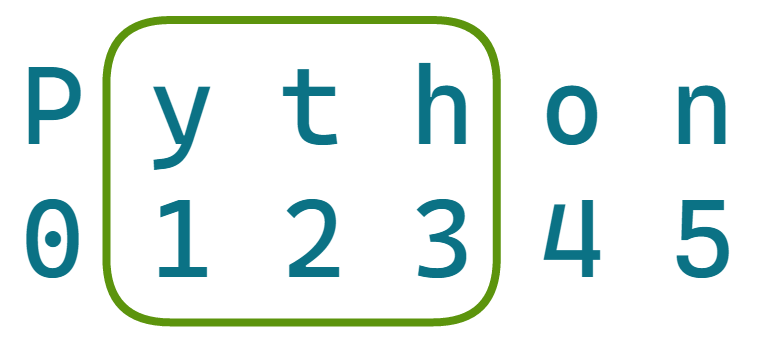
>>> string_1 = 'Python'
>>> string_1[1:4]
'yth'
While you use the begin
worth, the usual begin
The worth zero is used, and the phase begins at index zero and extends to cease - 1
.
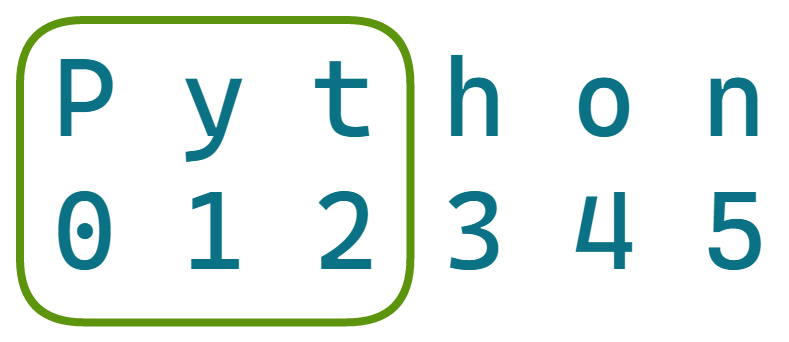
right here the cease
worth is 3, so the phase begins at index 0 and goes as much as index 2.
>>> string_1[:3]
'Pyt'
While you use the cease
index, you see that the phase begins from the begin
index(1) and extends to the tip of the string.
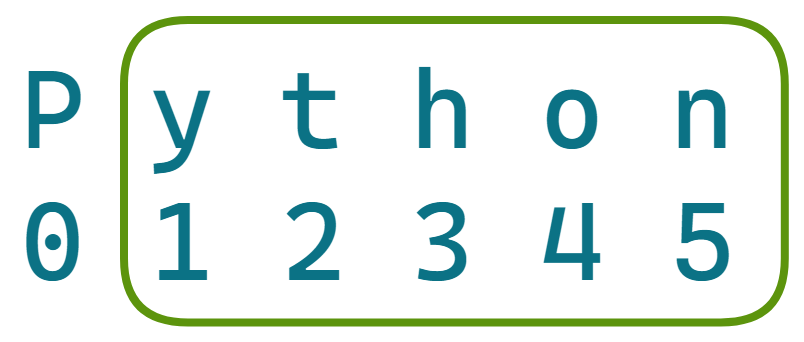
>>> string_1[1:]
'ython'
Ignoring each the begin
and the cease
values returns a part of the complete string.
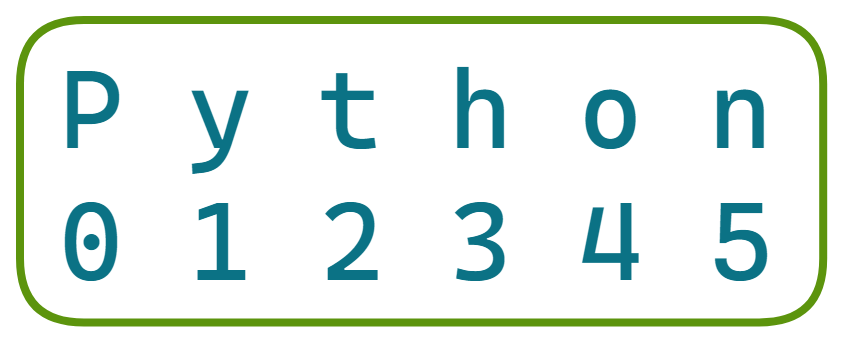
>>> string_1[::]
'Python'
Let’s create a phase with the step
worth. Set the begin
, cease
And step
values at 1, 5, and a pair of, respectively. We get a portion of the string that begins at 1 and extends to 4 (excluding the endpoint 5). each second character.
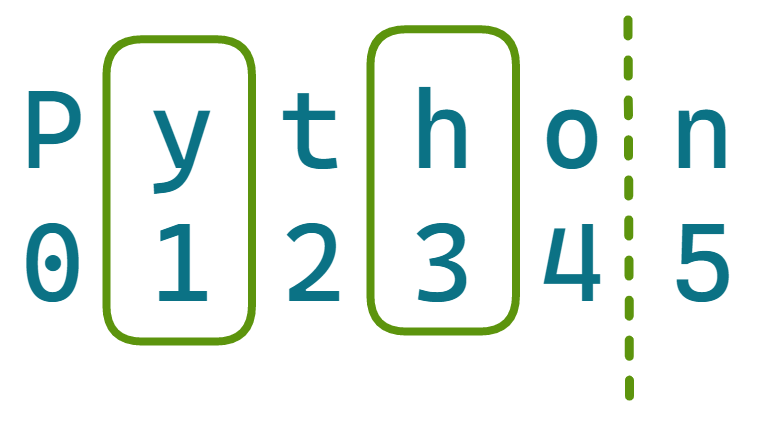
>>> string_1[1:5:2]
'yh'
While you use a adverse step, you may get a phase that begins on the finish of the sequence. With the step set to -2, string_1[5:2:-2]
returns the next phase:
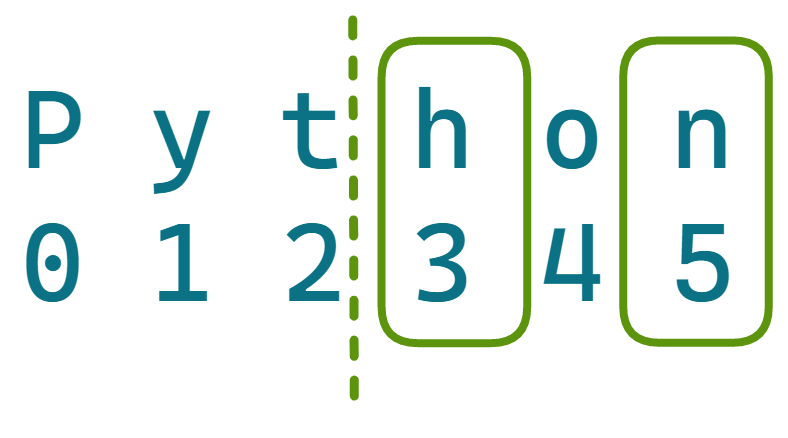
>>> string_1[5:2:-2]
'nh'
So to get a reverse copy of the string, we hit the begin
And cease
values and set the step to -1, as proven:
>>> string_1[::-1]
'nohtyP'
In abstract:
string[::-1]
returns a reversed copy of the string.
Reverse strings utilizing built-in features and string strategies
The built-in reversed()
perform in Python returns an inverted iterator over the weather of the string.
>>> string_1 = 'Python'
>>> reversed(string_1)
<reversed object at 0x00BEAF70>
So you’ll be able to undergo the reverse iterator utilizing a for loop:
for char in reversed(string_1):
print(char)
And entry the weather of the string within the reverse order.
# Output
n
o
h
t
y
P
You may then name the be part of()
methodology on the reverse iterator with the syntax: <sep>.be part of(reversed(some-string))
.
The code snippet beneath exhibits some examples the place the delimiter is a hyphen and a white area respectively.
>>> '-'.be part of(reversed(string1))
'n-o-h-t-y-P'
>>> ' '.be part of(reversed(string1))
'n o h t y P'
Right here we do not need a separator; so set the delimiter to an empty string to get a reversed copy of the string:
>>> ''.be part of(reversed(string1))
'nohtyP'
Utilizing
''.be part of(reversed(some-string))
returns a reversed copy of the string.
Evaluating execution occasions with timeit
Up to now we have realized two approaches to reversing Python strings. However which ones is quicker? Let’s discover out.
In an earlier instance the place we timed easy Python expressions, we had none setup
code. Right here we invert the Python sequence. Whereas the string reversal is executed for the variety of occasions specified by quantity
the setup
code is the initialization of the string that’s executed solely as soon as.
>>> import timeit
>>> timeit.timeit(stmt = 'string_1[::-1]', setup = "string_1 = 'Python'", quantity = 100000)
0.04951830000001678
>>> timeit.timeit(stmt = "''.be part of(reversed(string_1))", setup = "string_1 = 'Python'", quantity = 100000)
0.12858760000000302
For a similar variety of runs for reversing the given string, the slicing strings method is quicker than utilizing the be part of()
methodology and reversed()
perform.
Timing Python features utilizing timeit
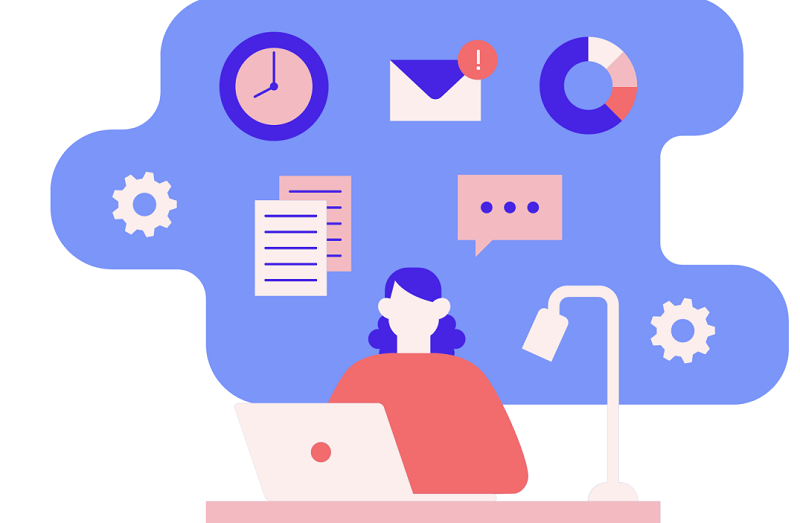
On this part, let’s discover ways to time Python features utilizing the timeit perform. Given an inventory of strings, the next perform hasDigit
returns the listing of strings containing at the very least one digit.
def hasDigit(somelist):
str_with_digit = []
for string in somelist:
check_char = [char.isdigit() for char in string]
if any(check_char):
str_with_digit.append(string)
return str_with_digit
Now we wish to measure the execution time of this Python perform hasDigit()
utilizing timeit
.
First, let’s determine the instruction to be timed (stmt
). It’s the name to the perform hasDigit()
with an inventory of strings as an argument. Subsequent, let’s do the arrange code. Are you able to guess what the setup
code needs to be?
To efficiently execute the perform name, the set up code should include:
- The definition of the perform
hasDigit()
- The initialization of the string argument listing
Let’s outline the set up code within the setup
string, as proven beneath:
setup = """
def hasDigit(somelist):
str_with_digit = []
for string in somelist:
check_char = [char.isdigit() for char in string]
if any(check_char):
str_with_digit.append(string)
return str_with_digit
thislist=['puffin3','7frost','blue']
"""
Then we will use the timeit
perform and get the execution time of the hasDigit()
perform for 100,000 runs.
import timeit
timeit.timeit('hasDigit(thislist)',setup=setup,quantity=100000)
# Output
0.2810094920000097
Conclusion
You have got realized how you can use it Python’s timeit perform to time expressions, features, and different callables. This might help you benchmark your code, evaluate the execution occasions of various implementations of the identical perform, and extra.
Let’s have a look at what we realized on this tutorial. You should utilize the timeit()
perform with the syntax timeit.timeit(stmt=...,setup=...,quantity=...)
. Alternatively, you’ll be able to run timeit on the command line to time quick code snippets.
As a subsequent step, you’ll be able to discover how you should utilize different Python profiling packages, similar to line-profiler and memprofiler, to profile your code for time and reminiscence, respectively.
Subsequent, discover ways to calculate the time distinction in Python.