An API or Software Programming Interface is a necessary a part of most software program architectures. It paves the way in which for various software program or providers to speak with one another effectively.
APIs present a way of abstraction by exposing some required performance and abstracting delicate or non-public knowledge. With authentication and authorization, you’ll be able to safe your APIs towards unauthorized entry or manipulation of knowledge.
Software program builders use numerous APIs to construct, check and optimize software program programs in a structured means. It permits them to speak with a software program system by way of a set of predefined guidelines or protocols.
What are API Gateways?
In a software program system, normally there is not only a single API that handles each service the software program gives; as a substitute, there are a variety of APIs that work collectively to ship knowledge to the consumer.
An API gateway, because the title suggests, acts as an entry level for numerous API requests and directs them to their particular API service or a microservice. This transfers the load of sending a number of API requests from the consumer to the API gateway, enhancing consumer efficiency.
For instance, a meals supply utility has an entire host of microservices for eating places, customers, provider rankings, supply associate rankings, routing algorithms, map service, and far more. So it might make far more sense if the consumer, i.e. the end-user utility, requests a single API, after which that API gateway sends requests to related microservices.
One other nice profit you get from implementing an API gateway is safety. You’ll be able to arrange a number of authentication and authorization programs to forestall attackers from misusing assets.
Constructing blocks of an API structure
There are a number of constructing blocks of an API structure, a few of that are listed right here:
#1. API interface
An API interface clearly defines the strategies or functionalities that may be accessed, with out disclosing implementation particulars. It defines a algorithm and methodologies for use to retrieve or modify assets.
For instance, in a RESTful API, you’ve got HTTP strategies like GET, PUT, POST, DELETE, and many others. to work together with assets.
#2. Route controllers
Controllers play a key position in API gateways as they deal with all API visitors from a number of shoppers and route it to a related API service.
As well as, controllers may also carry out request validation, response dealing with, authentication, and many others.
#3. Knowledge entry fashions
Each useful resource in a database has a sure sort of construction or form, and it’s higher to predefine that construction for validation functions. It is usually generally known as a schedule. The payload coming from the consumer will be validated towards the schema after which added to the database.
It prevents invalid or manipulated knowledge from getting into the database.
Parts of an API structure
- API providers: These are providers that present entry to a specific useful resource or set of assets. A number of API providers are required in a large-scale utility. These providers are disconnected from one another and handle assets independently.
- Documentation: API documentation is critical for builders to grasp the efficient use of API and the strategies it exposes. The documentation could embrace a listing of endpoints, greatest practices, request codecs, error dealing with, and many others.
- Evaluation and monitoring: An analytics dashboard is a vital part because it gives metrics reminiscent of API visitors, error charges, and efficiency, amongst many different insights.
Frequent API design architectures
REST – Consultant state switch
REST is an API structure fashion that makes use of the HTTP protocol and permits stateless communication between the consumer and the server.
In REST, the assets are recognized by URLs, which have particular endpoints for every useful resource. REST depends on HTTP strategies like GET, PUT, POST,
and many others. to change and create assets. The APIs that implement the REST structure are generally known as RESTful APIs.
SOAP – Easy object entry protocol
SOAP is a messaging protocol based mostly on XML. Messages in SOAP are encoded in XML paperwork and will be transferred from a SOAP sender to a SOAP receiver. There could also be a number of providers by way of which the message could go earlier than reaching the recipient.
The principle distinction between SOAP and REST is that REST is an architectural design that depends on HTTP, however SOAP itself is a protocol that may use totally different underlying protocols reminiscent of HTTP, SMTP, and many others. The response knowledge format in SOAP is XML.
gRPC – Google Distant Process name
Distant Process Name (RPC) is a way the place a perform on a distant server is known as by a consumer as if it had been referred to as regionally. gRPC is an open supply framework developed by Google. It makes use of proto buffers (protocol buffers), which is a language unbiased means of writing and encoding structured knowledge.
The info in protobuffers is collected by a gRPC compiler, making it interoperable. For instance, if the consumer code is written in Java and the server code is written in Go, the information specified within the protobuffers is suitable with each languages.
ChartQL
GraphQL is an open-source question language and runtime for constructing APIs. It permits shoppers to entry a number of assets by reaching a single entry level or endpoint. A specific supply just isn’t tied to a specific endpoint. You get what you specify within the request question.
You should outline a strongly typed schema for a specific question and a resolver perform that may run on that question. To alter assets, there’s a change question that you could specify in GraphQL.
API Structure Implementation – Finest Practices
Irrespective of how effectively you design your API structure, if it fails throughout manufacturing, it is no use. It ought to ship outcomes in line with actual world situations. Listed below are some key practices for getting ready the API structure for manufacturing:
✅ Use API gateway
API gateway helps within the efficient routing of API queries. An API gateway may also present safety and validation.
✅ Run API checks
Earlier than launching, be certain that your API has undergone in depth useful, integration, and efficiency testing. Automated testing frameworks may help streamline this course of.
✅ Concentrate on scalability
Create a scalable API structure that may handle rising visitors demand. If you wish to dynamically change the variety of API cases based mostly on demand, think about using auto-scaling strategies.
✅ Select internet hosting correctly
Take into account internet hosting suppliers that provide scalable options to satisfy rising visitors and buyer demand. Take into account options like load balancing, autoscaling, and the flexibleness to allocate extra assets as wanted.
Ensure that the internet hosting supplier can match the efficiency specs of your API, particularly in periods of excessive demand. Additionally discover serverless choices if that matches your online business wants.
How do you select the proper API structure?
Choosing an API structure depends upon the next issues:
- Enterprise Necessities: Analyze the enterprise targets to be achieved with the API and perceive the applying stream.
- Utilization situations: Asking the query why you want an API within the first place will assist you to immensely. Determining totally different utilization situations may help you design or choose an API structure.
- Scalability: Once more, understanding the enterprise necessities and utilization situations will assist you to design a scalable API structure that additionally performs.
- Developer expertise: Ensure that the API structure is simple to grasp in order that the brand new built-in builders can perceive it simply with none effort.
- Safety: In all probability an important side of API structure is safety. Ensure that your API structure is safe sufficient and compliant with privateness legal guidelines.
Subsequent, we’ll discover studying assets to enhance your API structure design abilities.
Studying assets
#1. Mastering API Structure: Designing, working, and creating API-based programs
This e book helps you be taught the fundamentals of APIs and discover sensible methods to design, construct, and check APIs.
Instance | Product | Judgement | Value | |
---|---|---|---|---|
|
Mastering API Structure: Designing, working, and creating API-based programs |
$45.99 |
Purchase on Amazon |
Additionally, you will discover ways to function, configure and implement your API system. This e book covers all the pieces from API gateways, service mesh, safety, TLS, and OAuth2 to creating present programs.
#2. Software program Structure: REST API Design – The Full Information
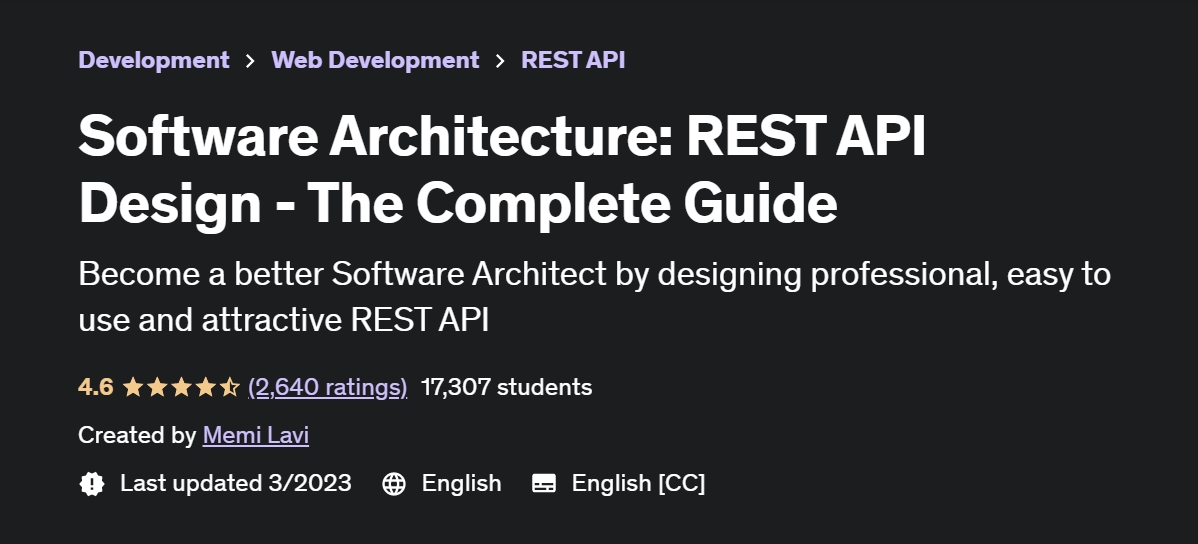
If you wish to be taught extra about RESTful APIs and the way they’re designed, this software program structure course is for you.
It consists of authentication, authorization, documenting REST APIs and numerous efficiency strategies to additional optimize your API design. The beauty of it’s that it covers the fundamentals of HTTP and the Postman API testing instrument.
#3. REST API design, growth and administration

Tutorials on numerous API administration platforms, reminiscent of Swagger, Apigee, and Mulesoft, are the primary spotlight of this course. This course is for individuals who wish to discover purposes of REST APIs and are concerned with designing them.
#4. Designing RESTful APIs: Learn to design an API from scratch

Making a REST API from scratch is what you will get on this RESTful API design course. Requests, responses, API design, and operations are some helpful matters lined. If you’re a newbie nonetheless studying the fundamentals of REST then I believe you need to go for it.
Final phrases
You’ll be able to select the perfect API structure in line with your online business and technical targets by contemplating integration wants, efficiency issues, safety necessities, and future scalability and extensibility.
Then jump-start your software program testing profession with these programs and assets.