In Python, probably the most in style methods to retailer sequential or ordered information is to make use of lists. An inventory in Python is an ordered, mutable, built-in information construction used to retailer a set of knowledge. Objects saved in an inventory are listed from scratch and customers can change the contents of an inventory after it’s created.
The truth that objects in an inventory are listed permits the storage of duplicate objects in an inventory. Lists in Python may also comprise parts of various information varieties. Objects in an inventory are separated by commas and enclosed in sq. brackets.
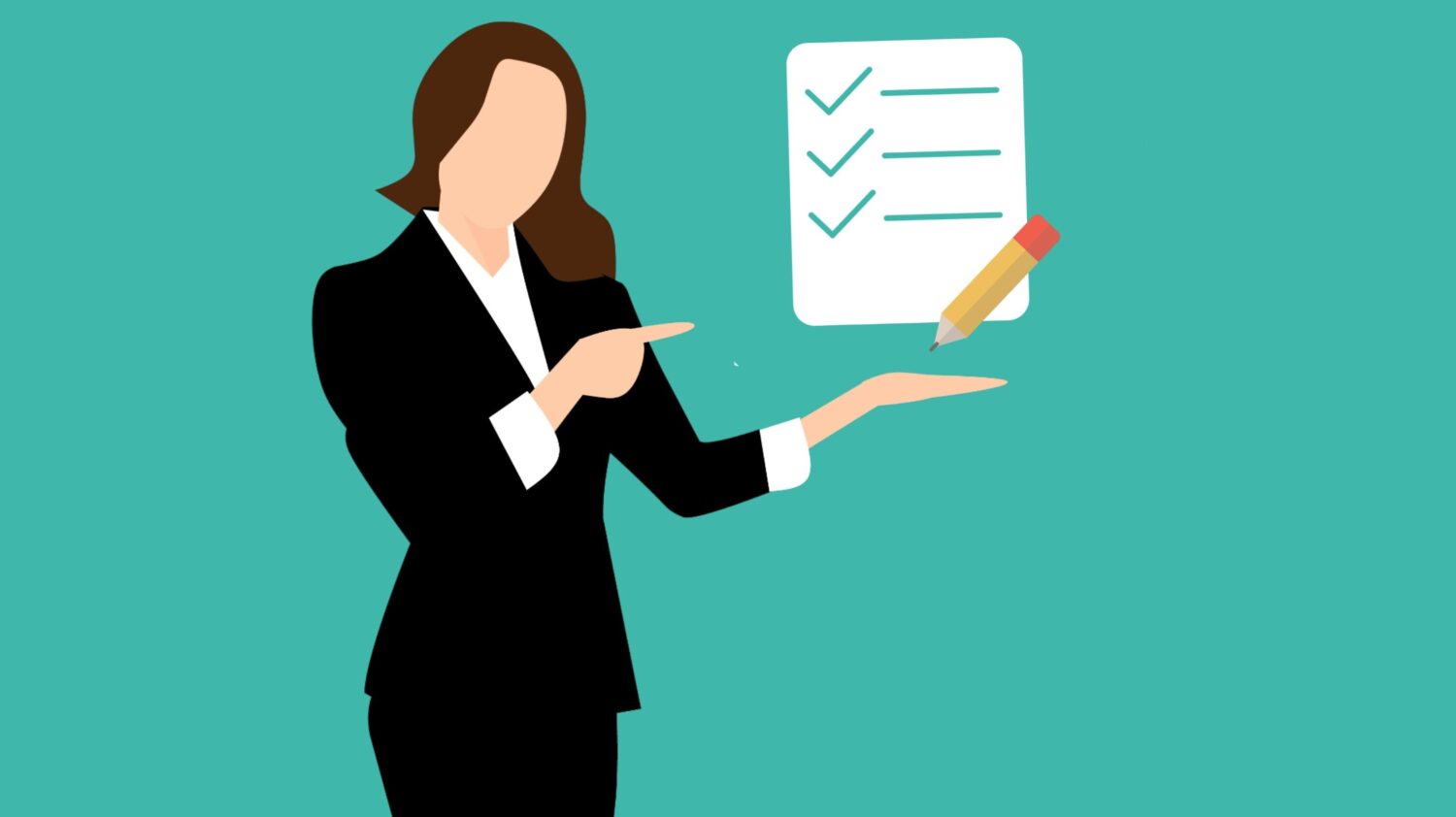
Listed here are examples of lists in Python:
# An inventory containing one information kind - String
colours = ['Red', 'Orange', 'Yellow', 'Green', 'Blue', 'Indigo', 'Violet']
# An inventory containing a number of information varieties
films = ['Transformers', 2012, 'Avengers', 300]
# An inventory with duplicate values
customers = ['John', 'Mary', 'Elizabeth', 'John']
Lists are a really versatile information construction and you’ll carry out many operations on the information saved in lists. A typical and helpful operation carried out on lists is filtering the information saved within the listing.
Why filtering lists is necessary
Filtering an inventory means extracting particular subsets of knowledge that meet a sure standards. For instance, we could solely have an interest within the even numbers in an inventory containing the numbers 1 via 10. To extract such information from the listing, we simply have to filter the listing to get numbers which are precisely divisible by two.
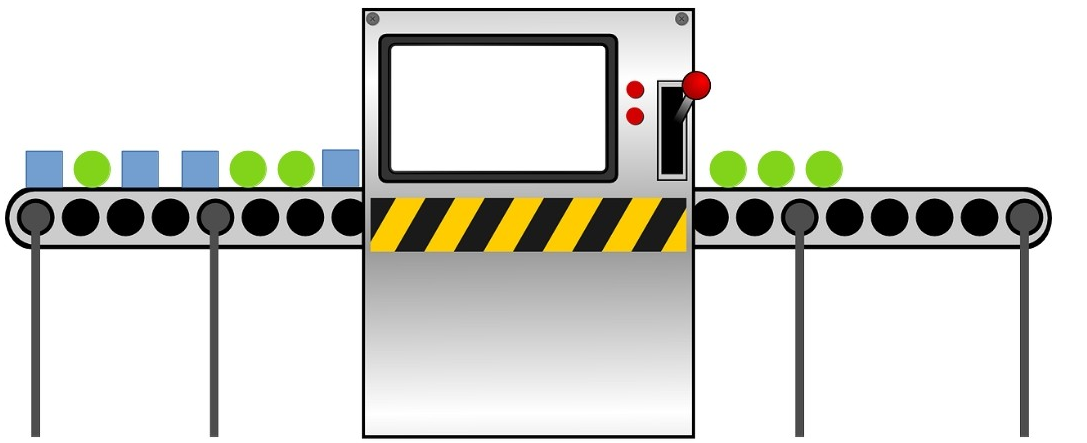
Filtering is particularly helpful in information manipulation and information evaluation as a result of it may take away undesirable objects from lists, create new lists with objects that meet sure standards, and extract information that meets sure circumstances.
Some sensible functions of filter lists embody:
- Knowledge validation – When working with an inventory, you could need to work solely with information that meets sure standards. For instance, in an inventory of customers, you is perhaps fascinated with customers with each a primary and final identify. Filtering permits you to confirm and validate the information within the listing earlier than processing it. This prevents you from working with information that doesn’t meet your necessities.
- Clear up information – As a result of lists can retailer a wide range of information varieties, it is not unusual for lists to comprise undesirable information that you just’re not fascinated with. For instance, in an inventory of names, you will not be fascinated with numeric values within the listing. Filtering permits you to take away the undesirable information.
- Knowledge evaluation – Knowledge filtering is especially helpful in information evaluation as a result of it permits you to deal with particular subsets of knowledge, making it simple to establish patterns and tendencies. For instance, in an inventory of scholars, you may filter female and male college students and use the lead to information evaluation. You’ll be able to even filter by age, location, and rank. This lets you draw extra helpful conclusions from particular subsets of knowledge in an inventory
Checklist filtering is a really helpful function as a result of it offers you management over the information saved in an inventory, so you may solely work with the information you have an interest in.
Focusing on particular subsets of knowledge saved in an inventory could be very helpful because it streamlines information processing and information evaluation making the processes higher, sooner and extra correct.
Utilizing the filter() operate
The filter() operate is a built-in Python operate that can be utilized to iterate via an iterable, similar to an inventory, tuples, set, or dictionary, and extract objects from the iterable that fulfill a given situation.
An Iterable in Python is an object that may be looped via its parts one after the other. Iterating via an iterable returns the objects within the iterable one after the other.
The syntax for the filter operate is as follows:
filter(operate, iterable)
operate – a Python operate that incorporates the filter situation
iterable – the iterable being filtered. On this case we use an inventory.
The filter() operate takes the handed operate and applies it to every merchandise within the handed iterable, testing the filter situation towards the merchandise. If the merchandise meets the situation, it returns the boolean worth of true, which is chosen by the filter() operate. If it doesn’t meet the situation, the merchandise is not going to be chosen.
The filter() operate returns an iterable containing objects that meet the filter situation. You’ll be able to create a brand new listing of things that match the filter situation through the use of the listing() operate.
To see the… filter() operate in motion, try the listing beneath, which is filtered to pick numbers lower than 50:
#An inventory of numbers
numbers = [79, 15, 92, 53, 46, 24, 81, 77, 37, 61]
# operate containing the filtering situation
def is_even(num):
if num < 50:
return True
else:
return False
# the operate listing() is used to create an inventory from the iterable
# returned by the filter() operate
filtered_numbers = listing(filter(is_even, numbers))
print(filtered_numbers)
The code above offers the next outcome:
[15, 46, 24, 37]
Utilizing a for loop
In Python, a for loop is a management stream assertion used to iterate via sequential information buildings similar to an inventory, tuples, strings, and arrays. A for loop repeatedly executes a block of code for every merchandise in a sequence.
The final syntax for a for loop is as follows:
for merchandise in iterable:
# Block of code to be executed for every merchandise within the iterabele
article – a variable that represents the present merchandise being processed in an iteration of the loop
iterable – the sequence over which the for loop iterates. On this case an inventory
To filter an inventory utilizing a for loop, we have to go our filter situation within the part for the code block to be executed. On this manner, every merchandise is evaluated to see if it meets a sure situation.
If you use a for loop to filter an inventory, you will need to additionally create an empty listing the place you add values that fulfill your filter situation.
To see this in motion, let’s filter an inventory of numbers to get numbers lower than 50 utilizing a for loop:
numbers = [79, 15, 92, 53, 46, 24, 81, 77, 37, 61]
filtered_numbers = []
for num in numbers:
if num < 50:
# append() used so as to add a quantity that passes the situation
# into filtered_numbers.
filtered_numbers.append(num)
print(filtered_numbers)
The code above offers the next outcome:
[15, 46, 24, 37]
Use a distinct listing
You’ll be able to filter an inventory towards one other listing by checking whether or not an merchandise in an inventory that you just need to filter is in one other listing. For instance, think about the next two lists
letters = ['a', 'h', 'q', 'd', 's', 'x', 'g', 'j', 'e', 'o', 'k', 'f', 'c', 'b', 'n']
vowels = ['a', 'e', 'i', 'o', 'u']
named within the listing letters above we are able to decide which objects within the listing aren’t vowels by checking if the merchandise is within the vowels listing or not. If an merchandise is just not within the vowels listing, then it’s not a vowel.
This manner we are able to get all of the characters in it letters these aren’t vowels. To do that, run the next code:
letters = ['a', 'h', 'q', 'd', 's', 'x', 'g', 'j', 'e', 'o', 'k', 'f', 'c', 'b', 'n']
vowels = ['a', 'e', 'i', 'o', 'u']
not_vowel = []
for letter in letters:
if letter not in vowels:
not_vowel.append(letter)
print(not_vowel)
The code above prints the next output, which incorporates characters in letters these aren’t vowels.
['h', 'q', 'd', 's', 'x', 'g', 'j', 'k', 'f', 'c', 'b', 'n']
Use listing comprehension
In Python, listing comprehension offers a shorter, concise, and cleaner syntax for creating a brand new listing from an present listing. Checklist comprehension has the flexibility to cut back the a number of traces of code used to create a brand new listing from one other listing to a single line utilizing a for loop.
It’s because whenever you use listing comprehension, you outline objects on one line and add them to the brand new listing.
The syntax for understanding lists is as follows:
new_list = [expression for element in iterable if condition]
new listing – a brand new listing of parts added by the listing comprehension
expression – An operation utilized to every merchandise of the iterable
article – variable identify representing the at the moment lively merchandise within the iterable
iterable – an iterable from which objects are chosen.
as a situation – an non-obligatory element the place a situation may be added to filter objects in order that solely objects that meet a sure situation are added to the brand new listing that’s created.
To see the understanding of lists in motion and the way a lot it may simplify the filtering course of and creating a brand new listing, we use the letters And vowels listing to filter objects from the mail listing that aren’t within the listing vowels listing. To do that, run the next code:
letters = ['a', 'h', 'q', 'd', 's', 'x', 'g', 'j', 'e', 'o', 'k', 'f', 'c', 'b', 'n']
vowels = ['a', 'e', 'i', 'o', 'u']
# listing comprehension
not_vowel = [letter for letter in letters if letter not in vowels]
print(not_vowel)
The output of the above code is proven beneath:
['h', 'q', 'd', 's', 'x', 'g', 'j', 'k', 'f', 'c', 'b', 'n']
The output is just like the earlier instance, the place a for loop was used to filter one listing towards one other listing. Nonetheless, fewer traces of code are used to realize the identical outcome.
Conclusion
When working with information in Python, a typical operation you want to carry out is filtering information from an iterable file, similar to an inventory. It is a very helpful step in information evaluation and information processing because it permits you to work solely with information that’s related to the operation or processing being carried out.
A very talked-about sequential information construction in Python is the listing. If you end up working with an inventory and have to filter it, think about using one of many strategies highlighted within the article.
You can even examine utilizing lambda features in Python with examples.